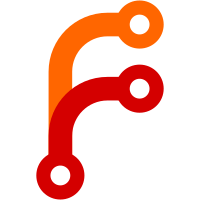
scene management code and organizing it within simgear. My strategy is to identify the code I want to move, and break it's direct flightgear dependencies. Then it will be free to move over into the simgear package. - Moved some property specific code into simgear/props/ - Split out the condition code from fgfs/src/Main/fg_props and put it in it's own source file in simgear/props/ - Created a scene subdirectory for scenery, model, and material property related code. - Moved location.[ch]xx into simgear/scene/model/ - The location and condition code had dependencies on flightgear's global state (all the globals-> stuff, the flightgear property tree, etc.) SimGear code can't depend on it so that data has to be passed as parameters to the functions/methods/constructors. - This need to pass data as function parameters had a dramatic cascading effect throughout the FlightGear code.
138 lines
3.9 KiB
C++
138 lines
3.9 KiB
C++
/**************************************************************************
|
|
* gui.cxx
|
|
*
|
|
* Written 1998 by Durk Talsma, started Juni, 1998. For the flight gear
|
|
* project.
|
|
*
|
|
* Additional mouse supported added by David Megginson, 1999.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of the
|
|
* License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
*
|
|
* $Id$
|
|
**************************************************************************/
|
|
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
# include <config.h>
|
|
#endif
|
|
|
|
#include <simgear/compiler.h>
|
|
|
|
#ifdef HAVE_WINDOWS_H
|
|
# include <windows.h>
|
|
#endif
|
|
|
|
#include <simgear/misc/exception.hxx>
|
|
#include <simgear/misc/sg_path.hxx>
|
|
#include <simgear/props/props.hxx>
|
|
#include <simgear/props/props_io.hxx>
|
|
|
|
#include <plib/pu.h>
|
|
|
|
#include <Include/general.hxx>
|
|
#include <Main/globals.hxx>
|
|
#include <Main/fg_props.hxx>
|
|
|
|
#include "gui.h"
|
|
#include "gui_local.hxx"
|
|
#include "net_dlg.hxx"
|
|
#include "preset_dlg.hxx"
|
|
|
|
|
|
// main.cxx hack, should come from an include someplace
|
|
extern void fgInitVisuals( void );
|
|
extern void fgRenderFrame( void );
|
|
|
|
extern void initDialog (void);
|
|
extern void mkDialogInit (void);
|
|
extern void ConfirmExitDialogInit(void);
|
|
|
|
|
|
puFont guiFnt = 0;
|
|
fntTexFont *guiFntHandle = 0;
|
|
|
|
|
|
/* -------------------------------------------------------------------------
|
|
init the gui
|
|
_____________________________________________________________________*/
|
|
|
|
|
|
void guiInit()
|
|
{
|
|
char *mesa_win_state;
|
|
|
|
// Initialize PUI
|
|
puInit();
|
|
puSetDefaultStyle ( PUSTYLE_SMALL_BEVELLED ); //PUSTYLE_DEFAULT
|
|
puSetDefaultColourScheme (0.8, 0.8, 0.9, 0.8);
|
|
|
|
initDialog();
|
|
|
|
// Next check home directory
|
|
SGPath fntpath;
|
|
char* envp = ::getenv( "FG_FONTS" );
|
|
if ( envp != NULL ) {
|
|
fntpath.set( envp );
|
|
} else {
|
|
fntpath.set( globals->get_fg_root() );
|
|
fntpath.append( "Fonts" );
|
|
}
|
|
|
|
// Install our fast fonts
|
|
SGPropertyNode *locale = globals->get_locale();
|
|
fntpath.append(locale->getStringValue("font", "typewriter.txf"));
|
|
guiFntHandle = new fntTexFont ;
|
|
guiFntHandle -> load ( (char *)fntpath.c_str() ) ;
|
|
puFont GuiFont ( guiFntHandle, 15 ) ;
|
|
puSetDefaultFonts( GuiFont, GuiFont ) ;
|
|
guiFnt = puGetDefaultLabelFont();
|
|
|
|
if (!fgHasNode("/sim/startup/mouse-pointer")) {
|
|
// no preference specified for mouse pointer, attempt to autodetect...
|
|
// Determine if we need to render the cursor, or if the windowing
|
|
// system will do it. First test if we are rendering with glide.
|
|
if ( strstr ( general.get_glRenderer(), "Glide" ) ) {
|
|
// Test for the MESA_GLX_FX env variable
|
|
if ( (mesa_win_state = getenv( "MESA_GLX_FX" )) != NULL) {
|
|
// test if we are fullscreen mesa/glide
|
|
if ( (mesa_win_state[0] == 'f') ||
|
|
(mesa_win_state[0] == 'F') ) {
|
|
puShowCursor ();
|
|
}
|
|
}
|
|
}
|
|
// mouse_active = ~mouse_active;
|
|
} else if ( !fgGetBool("/sim/startup/mouse-pointer") ) {
|
|
// don't show pointer
|
|
} else {
|
|
// force showing pointer
|
|
puShowCursor();
|
|
// mouse_active = ~mouse_active;
|
|
}
|
|
|
|
// MOUSE_VIEW mode stuff
|
|
initMouseQuat();
|
|
|
|
// Set up our Dialog Boxes
|
|
ConfirmExitDialogInit();
|
|
fgPresetInit();
|
|
|
|
#ifdef FG_NETWORK_OLK
|
|
NewNetIdInit();
|
|
NewNetFGDInit();
|
|
#endif
|
|
|
|
mkDialogInit();
|
|
}
|