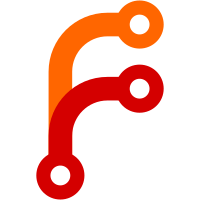
system. A chap from Germany called Alexander Kappes (cc'd) got in touch with me a few weeks ago and has written the start of Approach control. At the moment tuning in to a valid approach frequency (Dortmund or East Midlands) should result in vectors to a spot about 3 miles from the active runway, and a telling off if you stray too far from the correct course, in the console window. He seems to know what he's doing so expect this to improve rapidly!! I've added a rudimentry AI manager and a hardwired Cessna at KEMT on the runway - I'll remove it before the next release if I don't have it flying by then. There seems to be an issue with framerate which drops alarmingly when looking at it - I've a feeling that I've possibly created several Cessnas on top of each other, but am not sure.
100 lines
3.1 KiB
C++
100 lines
3.1 KiB
C++
// FGAILocalTraffic - AIEntity derived class with enough logic to
|
|
// fly and interact with the traffic pattern.
|
|
//
|
|
// Written by David Luff, started March 2002.
|
|
//
|
|
// Copyright (C) 2002 David C. Luff - david.luff@nottingham.ac.uk
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of the GNU General Public License as
|
|
// published by the Free Software Foundation; either version 2 of the
|
|
// License, or (at your option) any later version.
|
|
//
|
|
// This program is distributed in the hope that it will be useful, but
|
|
// WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU General Public License
|
|
// along with this program; if not, write to the Free Software
|
|
// Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
|
|
/*****************************************************************
|
|
*
|
|
* WARNING - Curt has some ideas about AI traffic so anything in here
|
|
* may get rewritten or scrapped. Contact Curt curt@flightgear.org
|
|
* before spending any time or effort on this code!!!
|
|
*
|
|
******************************************************************/
|
|
|
|
#include <Main/globals.hxx>
|
|
//#include <simgear/constants.h>
|
|
#include <simgear/math/point3d.hxx>
|
|
#include <simgear/math/sg_geodesy.hxx>
|
|
#include <simgear/misc/sg_path.hxx>
|
|
#include <string>
|
|
|
|
SG_USING_STD(string);
|
|
|
|
#include "ATCmgr.hxx"
|
|
#include "AILocalTraffic.hxx"
|
|
|
|
FGAILocalTraffic::FGAILocalTraffic() {
|
|
}
|
|
|
|
FGAILocalTraffic::~FGAILocalTraffic() {
|
|
}
|
|
|
|
void FGAILocalTraffic::Init() {
|
|
// Hack alert - Hardwired path!!
|
|
string planepath = "Aircraft/c172/Models/c172-dpm.ac";
|
|
SGPath path = globals->get_fg_root();
|
|
path.append(planepath);
|
|
ssgTexturePath((char*)path.dir().c_str());
|
|
model = ssgLoad((char*)planepath.c_str());
|
|
if (model == 0) {
|
|
model = ssgLoad((char*)"Models/Geometry/glider.ac");
|
|
if (model == 0)
|
|
cout << "Failed to load an aircraft model in AILocalTraffic\n";
|
|
} else {
|
|
cout << "AILocal Traffic Model loaded successfully\n";
|
|
}
|
|
position = new ssgTransform;
|
|
position->addKid(model);
|
|
|
|
// Hardwire to KEMT
|
|
lat = 34.081358;
|
|
lon = -118.037483;
|
|
elev = (287.0 + 0.5) * SG_FEET_TO_METER; // Ground is 296 so this should be above it
|
|
mag_hdg = -10.0;
|
|
pitch = 0.0;
|
|
roll = 0.0;
|
|
mag_var = -14.0;
|
|
|
|
// Activate the tower - this is dependent on the ATC system being initialised before the AI system
|
|
AirportATC a;
|
|
if(globals->get_ATC_mgr()->GetAirportATCDetails((string)"KEMT", &a)) {
|
|
if(a.tower_freq) { // Has a tower
|
|
tower = (FGTower*)globals->get_ATC_mgr()->GetATCPointer((string)"KEMT", TOWER); // Maybe need some error checking here
|
|
} else {
|
|
// Check CTAF, unicom etc
|
|
}
|
|
} else {
|
|
cout << "Unable to find airport details in FGAILocalTraffic::Init()\n";
|
|
}
|
|
|
|
Transform();
|
|
}
|
|
|
|
// Run the internal calculations
|
|
void FGAILocalTraffic::Update() {
|
|
hdg = mag_hdg + mag_var;
|
|
|
|
// This should become if(the plane has moved) then Transform()
|
|
static int i = 0;
|
|
if(i == 60) {
|
|
Transform();
|
|
i = 0;
|
|
}
|
|
i++;
|
|
}
|