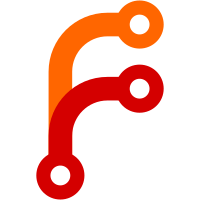
This patch removes some useless indirection when creating AIModels. It obsolets AIScenario*. AIEntities are just an intermediate copy of an other intermediate copy of an xml file on the way from the ai scenario configuration file to the AIModels. As such the AImodels can now be created directly from the property tree read from the scenario file. This reduces the amount of work needed to add an other AIModel and reduces the amount of copy operations done during initialization. It also moves internal knowledge of special AI models into these special AI models class instead of spreading that into the whole AIModel subdirectory which in turn enables to use carrier internal data structures for carrier internal data ... Also some unused variables are removed from the AIModel classes. I believe that there are still more of them, but that is what I stumbled accross ... Tested, like the other splitouts these days in a seperate tree and using the autopilot for some time, and in this case with a carrier start ...
65 lines
1.9 KiB
C++
65 lines
1.9 KiB
C++
// FGAIThermal - AIBase derived class creates an AI thunderstorm
|
|
//
|
|
// Written by David Culp, started Feb 2004.
|
|
//
|
|
// Copyright (C) 2004 David P. Culp - davidculp2@comcast.net
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of the GNU General Public License as
|
|
// published by the Free Software Foundation; either version 2 of the
|
|
// License, or (at your option) any later version.
|
|
//
|
|
// This program is distributed in the hope that it will be useful, but
|
|
// WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU General Public License
|
|
// along with this program; if not, write to the Free Software
|
|
// Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
|
|
#ifndef _FG_AIThermal_HXX
|
|
#define _FG_AIThermal_HXX
|
|
|
|
#include "AIManager.hxx"
|
|
#include "AIBase.hxx"
|
|
|
|
#include <string>
|
|
SG_USING_STD(string);
|
|
|
|
|
|
class FGAIThermal : public FGAIBase {
|
|
|
|
public:
|
|
|
|
FGAIThermal();
|
|
~FGAIThermal();
|
|
|
|
void readFromScenario(SGPropertyNode* scFileNode);
|
|
|
|
virtual bool init();
|
|
virtual void bind();
|
|
virtual void unbind();
|
|
virtual void update(double dt);
|
|
|
|
inline void setMaxStrength( double s ) { max_strength = s; };
|
|
inline void setDiameter( double d ) { diameter = d; };
|
|
inline void setHeight( double h ) { height = h; };
|
|
inline double getStrength() const { return strength; };
|
|
inline double getDiameter() const { return diameter; };
|
|
inline double getHeight() const { return height; };
|
|
|
|
virtual const char* getTypeString(void) const { return "thermal"; }
|
|
private:
|
|
|
|
void Run(double dt);
|
|
double max_strength;
|
|
double strength;
|
|
double diameter;
|
|
double height;
|
|
double factor;
|
|
};
|
|
|
|
|
|
|
|
#endif // _FG_AIThermal_HXX
|