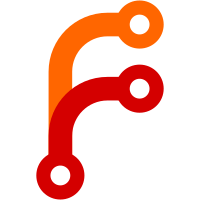
Partition depth in CameraGroup: Remove the ViewPartionNode scenegraph node. The split rendering of the scene, done to avoid Z buffer precision problems, is now done by two slave cameras of the viewer. Rename FGManipulator to FGEventHandler. Remove virtual member functions that aren't required for event handlers. Begin using camera group properties to update cameras at runtime; Initially only the viewport properties are used. When no camera group is found in the property tree (the default), create the properties for one. Expose the default window by name. Add a test for Boost headers to configure.ac. Boost is now a dependency. Remove GLUT and SDL versions of the OSG graphics.
129 lines
3 KiB
C++
129 lines
3 KiB
C++
#ifndef FGEVENTHANDLER_H
|
|
#define FGEVENTHANDLER_H 1
|
|
|
|
#include <map>
|
|
#include <osg/Quat>
|
|
#include <osgGA/GUIEventHandler>
|
|
#include <osgViewer/ViewerEventHandlers>
|
|
|
|
#include "fg_os.hxx"
|
|
|
|
namespace flightgear
|
|
{
|
|
class FGEventHandler : public osgGA::GUIEventHandler {
|
|
public:
|
|
FGEventHandler();
|
|
|
|
virtual ~FGEventHandler() {}
|
|
|
|
virtual const char* className() const {return "FGEventHandler"; }
|
|
#if 0
|
|
virtual void init(const osgGA::GUIEventAdapter& ea,
|
|
osgGA::GUIActionAdapter& us);
|
|
#endif
|
|
virtual bool handle(const osgGA::GUIEventAdapter& ea,
|
|
osgGA::GUIActionAdapter& us);
|
|
|
|
void setIdleHandler(fgIdleHandler idleHandler)
|
|
{
|
|
this->idleHandler = idleHandler;
|
|
}
|
|
|
|
fgIdleHandler getIdleHandler() const
|
|
{
|
|
return idleHandler;
|
|
}
|
|
|
|
void setDrawHandler(fgDrawHandler drawHandler)
|
|
{
|
|
this->drawHandler = drawHandler;
|
|
}
|
|
|
|
fgDrawHandler getDrawHandler() const
|
|
{
|
|
return drawHandler;
|
|
}
|
|
|
|
void setWindowResizeHandler(fgWindowResizeHandler windowResizeHandler)
|
|
{
|
|
this->windowResizeHandler = windowResizeHandler;
|
|
}
|
|
|
|
fgWindowResizeHandler getWindowResizeHandler() const
|
|
{
|
|
return windowResizeHandler;
|
|
}
|
|
|
|
void setKeyHandler(fgKeyHandler keyHandler)
|
|
{
|
|
this->keyHandler = keyHandler;
|
|
}
|
|
|
|
fgKeyHandler getKeyHandler() const
|
|
{
|
|
return keyHandler;
|
|
}
|
|
|
|
void setMouseClickHandler(fgMouseClickHandler mouseClickHandler)
|
|
{
|
|
this->mouseClickHandler = mouseClickHandler;
|
|
}
|
|
|
|
fgMouseClickHandler getMouseClickHandler()
|
|
{
|
|
return mouseClickHandler;
|
|
}
|
|
|
|
void setMouseMotionHandler(fgMouseMotionHandler mouseMotionHandler)
|
|
{
|
|
this->mouseMotionHandler = mouseMotionHandler;
|
|
}
|
|
|
|
fgMouseMotionHandler getMouseMotionHandler()
|
|
{
|
|
return mouseMotionHandler;
|
|
}
|
|
|
|
int getCurrentModifiers() const
|
|
{
|
|
return currentModifiers;
|
|
}
|
|
|
|
void setMouseWarped()
|
|
{
|
|
mouseWarped = true;
|
|
}
|
|
|
|
/** Whether or not resizing is supported. It might not be when
|
|
* using multiple displays.
|
|
*/
|
|
bool getResizable() { return resizable; }
|
|
void setResizable(bool _resizable) { resizable = _resizable; }
|
|
|
|
protected:
|
|
osg::ref_ptr<osg::Node> _node;
|
|
fgIdleHandler idleHandler;
|
|
fgDrawHandler drawHandler;
|
|
fgWindowResizeHandler windowResizeHandler;
|
|
fgKeyHandler keyHandler;
|
|
fgMouseClickHandler mouseClickHandler;
|
|
fgMouseMotionHandler mouseMotionHandler;
|
|
osg::ref_ptr<osgViewer::StatsHandler> statsHandler;
|
|
osg::ref_ptr<osgGA::GUIEventAdapter> statsEvent;
|
|
int statsType;
|
|
int currentModifiers;
|
|
std::map<int, int> numlockKeyMap;
|
|
void handleKey(const osgGA::GUIEventAdapter& ea, int& key, int& modifiers);
|
|
bool resizable;
|
|
bool mouseWarped;
|
|
// workaround for osgViewer double scroll events
|
|
bool scrollButtonPressed;
|
|
int release_keys[128];
|
|
void handleStats(osgGA::GUIActionAdapter& us);
|
|
};
|
|
|
|
void eventToWindowCoords(const osgGA::GUIEventAdapter* ea, double& x, double& y);
|
|
void eventToWindowCoordsYDown(const osgGA::GUIEventAdapter* ea,
|
|
double& x, double& y);
|
|
}
|
|
#endif
|