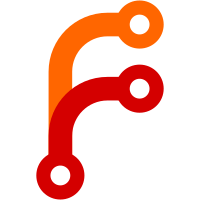
- Rename fatalMessageBox() to fatalMessageBoxWithoutExit(). This should prevent the kind of bug that prompted this set of changes: someone calling fatalMessageBox(), assuming the program would stop at that point, whereas in reality it did not. - Add new function fatalMessageBoxThenExit(). This is not vital of course, but allows one to spare one line here and there and to apply the DRY principle for such fatal exits. - Replace every existing call to fatalMessageBox() with one or the other of the two new functions. Improve formatting along the way. This fixes a few bugs of the kind explained above.
35 lines
753 B
C++
35 lines
753 B
C++
#ifndef FG_GUI_MESSAGE_BOX_HXX
|
|
#define FG_GUI_MESSAGE_BOX_HXX
|
|
|
|
#include <string>
|
|
#include <cstdlib>
|
|
|
|
namespace flightgear
|
|
{
|
|
|
|
enum MessageBoxResult
|
|
{
|
|
MSG_BOX_OK,
|
|
MSG_BOX_YES,
|
|
MSG_BOX_NO
|
|
};
|
|
|
|
MessageBoxResult modalMessageBox(const std::string& caption,
|
|
const std::string& msg,
|
|
const std::string& moreText = std::string());
|
|
|
|
MessageBoxResult fatalMessageBoxWithoutExit(
|
|
const std::string& caption,
|
|
const std::string& msg,
|
|
const std::string& moreText = std::string());
|
|
|
|
[[noreturn]] void fatalMessageBoxThenExit(
|
|
const std::string& caption,
|
|
const std::string& msg,
|
|
const std::string& moreText = std::string(),
|
|
int exitStatus = EXIT_FAILURE);
|
|
|
|
|
|
} // of namespace flightgear
|
|
|
|
#endif // of FG_GUI_MESSAGE_BOX_HXX
|