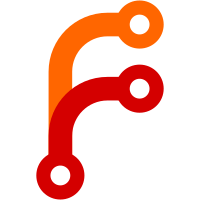
All necessary elements for an ADF gauge had been migrated from Cockpit/kr_87.cxx to Instrumentation/adf.cxx. Migrating the sound related elements was apparently planned, but not done yet. This intermediate state broke the ident morse sound: it couldn't get turned off and it always indicated "SF", regardless of the tuned-in frequency. The following patches continue the migration: adf-radio.diff => Base/Aircraft/Instruments/adf-radio.xml: --------------------------------------------------------------- * sets maximum volume to 1 (rather than 2); Not only is 1 loud enough (and 2 unpleasantly noisy), it also prevents the knob from being turned to non-existant positions. :-) * fixes wrong use of /instrumentation/adf/ident * the voice/ident selector(?) remains unchanged, but as it's not switched to "IDENT", there'll be no ident sound by default this is consistent with other sounds and DME. radiostack.diff => src/Cockpit/radiostack.[ch]xx: --------------------------------------------------------------- * comment out use of FGKR_87 class. kr_87.[ch]xx is now no longer used. kr-87adf.xml would no longer work, either, but isn't used anywhere, anyway. Future adf radios have to use the adf instrument, using xml/Nasal for specific hardware implementation details. adf.diff => src/Instrumentation/adf.[ch]xx: --------------------------------------------------------------- * adds ident morse sound capability using two new input properties: - /instrumentation/adf/volume-norm (double) - /instrumentation/adf/ident-audible (bool)
131 lines
2.7 KiB
C++
131 lines
2.7 KiB
C++
// radiostack.cxx -- class to manage an instance of the radio stack
|
|
//
|
|
// Written by Curtis Olson, started April 2000.
|
|
//
|
|
// Copyright (C) 2000 Curtis L. Olson - curt@flightgear.org
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of the GNU General Public License as
|
|
// published by the Free Software Foundation; either version 2 of the
|
|
// License, or (at your option) any later version.
|
|
//
|
|
// This program is distributed in the hope that it will be useful, but
|
|
// WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU General Public License
|
|
// along with this program; if not, write to the Free Software
|
|
// Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
//
|
|
// $Id$
|
|
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
# include <config.h>
|
|
#endif
|
|
|
|
#include <stdio.h> // snprintf
|
|
|
|
#include <simgear/compiler.h>
|
|
#include <simgear/math/sg_random.h>
|
|
|
|
#include <Aircraft/aircraft.hxx>
|
|
#include <Navaids/navlist.hxx>
|
|
|
|
#include "radiostack.hxx"
|
|
|
|
#include <string>
|
|
SG_USING_STD(string);
|
|
|
|
|
|
FGRadioStack *current_radiostack;
|
|
|
|
|
|
// Constructor
|
|
FGRadioStack::FGRadioStack() {
|
|
}
|
|
|
|
|
|
// Destructor
|
|
FGRadioStack::~FGRadioStack()
|
|
{
|
|
//adf.unbind();
|
|
beacon.unbind();
|
|
navcom1.unbind();
|
|
navcom2.unbind();
|
|
xponder.unbind();
|
|
}
|
|
|
|
|
|
void
|
|
FGRadioStack::init ()
|
|
{
|
|
navcom1.set_bind_index( 0 );
|
|
navcom1.init();
|
|
|
|
navcom2.set_bind_index( 1 );
|
|
navcom2.init();
|
|
|
|
//adf.init();
|
|
beacon.init();
|
|
xponder.init();
|
|
|
|
search();
|
|
update(0); // FIXME: use dt
|
|
|
|
// Search radio database once per second
|
|
globals->get_event_mgr()->addTask( "fgRadioSearch()", current_radiostack,
|
|
&FGRadioStack::search, 1 );
|
|
}
|
|
|
|
|
|
void
|
|
FGRadioStack::bind ()
|
|
{
|
|
//adf.bind();
|
|
beacon.bind();
|
|
dme.bind();
|
|
navcom1.set_bind_index( 0 );
|
|
navcom1.bind();
|
|
navcom2.set_bind_index( 1 );
|
|
navcom2.bind();
|
|
xponder.bind();
|
|
}
|
|
|
|
|
|
void
|
|
FGRadioStack::unbind ()
|
|
{
|
|
//adf.unbind();
|
|
beacon.unbind();
|
|
dme.unbind();
|
|
navcom1.unbind();
|
|
navcom2.unbind();
|
|
xponder.unbind();
|
|
}
|
|
|
|
|
|
// Update the various nav values based on position and valid tuned in navs
|
|
void
|
|
FGRadioStack::update(double dt)
|
|
{
|
|
//adf.update( dt );
|
|
beacon.update( dt );
|
|
navcom1.update( dt );
|
|
navcom2.update( dt );
|
|
dme.update( dt ); // dme is updated after the navcom's
|
|
xponder.update( dt );
|
|
}
|
|
|
|
|
|
// Update current nav/adf radio stations based on current postition
|
|
void FGRadioStack::search()
|
|
{
|
|
//adf.search();
|
|
beacon.search();
|
|
navcom1.search();
|
|
navcom2.search();
|
|
dme.search();
|
|
xponder.search();
|
|
}
|