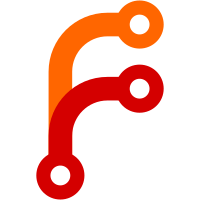
Stub in hooks for Propeller feathering controls and the turbo prop "condition" lever. I added a line in FGFDM.cpp to force control properties to exist if they don't already. This way you can specify anything you want and find them in the property browser, otherwise no one else may create them and you are stuck. In PropEngine::solve() the code original sets _running = true at the beginning and then sets running = false at the end. I changed this to save the current value at the start, set to true, solve(), and then restore the original value at the end. That way if we start off with _running = true, we don't have to hack up the calc() routine which wasn't using the value anyway. Finally I added some very initial support to shut down a turbine engine (_running = false) when the condition lever goes to zero.
51 lines
1.2 KiB
C++
51 lines
1.2 KiB
C++
#ifndef _TURBINEENGINE_HPP
|
|
#define _TURBINEENGINE_HPP
|
|
|
|
#include "Engine.hpp"
|
|
|
|
namespace yasim {
|
|
|
|
class TurbineEngine : public Engine {
|
|
public:
|
|
virtual TurbineEngine* isTurbineEngine() { return this; }
|
|
|
|
TurbineEngine(float power, float omega, float alt, float flatRating);
|
|
void setN2Range(float min, float max) { _n2Min = min; _n2Max = max; }
|
|
void setFuelConsumption(float bsfc) { _bsfc = bsfc; }
|
|
|
|
virtual void calc(float pressure, float temp, float speed);
|
|
virtual void stabilize();
|
|
virtual void integrate(float dt);
|
|
|
|
void setCondLever( float lever ) {
|
|
_cond_lever = lever;
|
|
}
|
|
virtual float getTorque() { return _torque; }
|
|
virtual float getFuelFlow() { return _fuelFlow; }
|
|
float getN2() { return _n2; }
|
|
|
|
private:
|
|
void setOutputFromN2();
|
|
|
|
float _cond_lever;
|
|
|
|
float _maxTorque;
|
|
float _flatRating;
|
|
float _rho0;
|
|
float _bsfc; // SI units! kg/s per watt
|
|
float _n2Min;
|
|
float _n2Max;
|
|
|
|
float _n2Target;
|
|
float _torqueTarget;
|
|
float _fuelFlowTarget;
|
|
|
|
float _n2;
|
|
float _rho;
|
|
float _omega;
|
|
float _torque;
|
|
float _fuelFlow;
|
|
};
|
|
|
|
}; // namespace yasim
|
|
#endif // _TURBINEENGINE_HPP
|