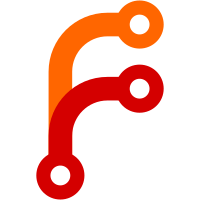
Partition depth in CameraGroup: Remove the ViewPartionNode scenegraph node. The split rendering of the scene, done to avoid Z buffer precision problems, is now done by two slave cameras of the viewer. Rename FGManipulator to FGEventHandler. Remove virtual member functions that aren't required for event handlers. Begin using camera group properties to update cameras at runtime; Initially only the viewport properties are used. When no camera group is found in the property tree (the default), create the properties for one. Expose the default window by name. Add a test for Boost headers to configure.ac. Boost is now a dependency. Remove GLUT and SDL versions of the OSG graphics.
97 lines
2.3 KiB
C++
97 lines
2.3 KiB
C++
|
|
#ifndef __FG_RENDERER_HXX
|
|
#define __FG_RENDERER_HXX 1
|
|
|
|
#include <simgear/screen/extensions.hxx>
|
|
#include <simgear/scene/util/SGPickCallback.hxx>
|
|
|
|
#include <osg/ref_ptr>
|
|
|
|
namespace osg
|
|
{
|
|
class Camera;
|
|
class Group;
|
|
}
|
|
|
|
namespace osgGA
|
|
{
|
|
class GUIEventAdapter;
|
|
}
|
|
|
|
namespace osgShadow
|
|
{
|
|
class ShadowedScene;
|
|
}
|
|
|
|
namespace osgViewer
|
|
{
|
|
class Viewer;
|
|
}
|
|
|
|
namespace flightgear
|
|
{
|
|
class FGEventHandler;
|
|
}
|
|
|
|
#define FG_ENABLE_MULTIPASS_CLOUDS 1
|
|
|
|
class SGSky;
|
|
extern SGSky *thesky;
|
|
|
|
extern glPointParameterfProc glPointParameterfPtr;
|
|
extern glPointParameterfvProc glPointParameterfvPtr;
|
|
extern bool glPointParameterIsSupported;
|
|
extern bool glPointSpriteIsSupported;
|
|
|
|
|
|
class FGRenderer {
|
|
|
|
public:
|
|
|
|
FGRenderer();
|
|
~FGRenderer();
|
|
|
|
void splashinit();
|
|
void init();
|
|
|
|
static void resize(int width, int height );
|
|
|
|
// calling update( refresh_camera_settings = false ) will not
|
|
// touch window or camera settings. This is useful for the tiled
|
|
// renderer which needs to set the view frustum itself.
|
|
static void update( bool refresh_camera_settings );
|
|
inline static void update() { update( true ); }
|
|
|
|
/** Set all the camera parameters at once. aspectRatio is height / width.
|
|
*/
|
|
static void setCameraParameters(float vfov, float aspectRatio,
|
|
float zNear, float zFar);
|
|
/** Just pick into the scene and return the pick callbacks on the way ...
|
|
*/
|
|
static bool pick( std::vector<SGSceneryPick>& pickList,
|
|
const osgGA::GUIEventAdapter* ea );
|
|
|
|
/** Get and set the OSG Viewer object, if any.
|
|
*/
|
|
osgViewer::Viewer* getViewer() { return viewer.get(); }
|
|
const osgViewer::Viewer* getViewer() const { return viewer.get(); }
|
|
void setViewer(osgViewer::Viewer* viewer);
|
|
/** Get and set the manipulator object, if any.
|
|
*/
|
|
flightgear::FGEventHandler* getEventHandler() { return eventHandler.get(); }
|
|
const flightgear::FGEventHandler* getEventHandler() const { return eventHandler.get(); }
|
|
void setEventHandler(flightgear::FGEventHandler* manipulator);
|
|
|
|
/** Add a top level camera.
|
|
*/
|
|
void addCamera(osg::Camera* camera, bool useSceneData);
|
|
|
|
protected:
|
|
osg::ref_ptr<osgViewer::Viewer> viewer;
|
|
osg::ref_ptr<flightgear::FGEventHandler> eventHandler;
|
|
};
|
|
|
|
bool fgDumpSceneGraphToFile(const char* filename);
|
|
bool fgDumpTerrainBranchToFile(const char* filename);
|
|
|
|
#endif
|