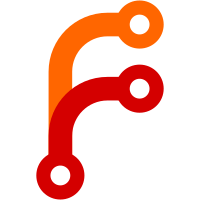
- changed FGSubsystem::update(int) to FGSubsystem::update(delta_time_sec); the argument is now delta time in seconds rather than milliseconds - added FGSubsystem::suspend(), FGSubsystem::suspend(bool), FGSubsystem::resume(), and FGSubsystem::is_suspended(), all with default implementations; is_suspended takes account of the master freeze as well as the subsystem's individual suspended state - the FDMs now use the delta time argument the same as the rest of FlightGear; formerly, main.cxx made a special case and passed a multiloop argument - FDMs now calculate multiloop internally instead of relying on main.cxx There are probably some problems -- I've done basic testing with the major FDMs and subsystems, but we'll probably need a few weeks to sniff out bugs.
93 lines
3.1 KiB
C++
93 lines
3.1 KiB
C++
// ATCdisplay.hxx - class to manage the graphical display of ATC messages.
|
|
// - The idea is to separate the display of ATC messages from their
|
|
// - generation so that the generation may come from any source.
|
|
//
|
|
// Written by David Luff, started October 2001.
|
|
//
|
|
// Copyright (C) 2001 David C Luff - david.luff@nottingham.ac.uk
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of the GNU General Public License as
|
|
// published by the Free Software Foundation; either version 2 of the
|
|
// License, or (at your option) any later version.
|
|
//
|
|
// This program is distributed in the hope that it will be useful, but
|
|
// WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU General Public License
|
|
// along with this program; if not, write to the Free Software
|
|
// Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
|
|
#ifndef _FG_ATC_DISPLAY_HXX
|
|
#define _FG_ATC_DISPLAY_HXX
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
# include <config.h>
|
|
#endif
|
|
|
|
#include <Main/fgfs.hxx>
|
|
|
|
#include <vector>
|
|
#include <string>
|
|
|
|
SG_USING_STD(vector);
|
|
SG_USING_STD(string);
|
|
|
|
struct atcMessage {
|
|
string msg;
|
|
bool repeating;
|
|
int counter; // count of how many iterations since posting
|
|
int start_count; // value of counter at which display should start
|
|
int stop_count; // value of counter at which display should stop
|
|
int id;
|
|
};
|
|
|
|
// ASSUMPTION - with two radios the list won't be long so we don't need to map the id's
|
|
typedef vector<atcMessage> atcMessageList;
|
|
typedef atcMessageList::iterator atcMessageListIterator;
|
|
|
|
class FGATCDisplay : public FGSubsystem
|
|
{
|
|
|
|
private:
|
|
bool rep_msg; // Flag to indicate there is a repeating transmission to display
|
|
bool change_msg_flag; // Flag to indicate that the repeating message has changed
|
|
float dsp_offset1; // Used to set the correct position of scrolling display
|
|
float dsp_offset2;
|
|
string rep_msg_str; // The repeating transmission to play
|
|
atcMessageList msgList;
|
|
atcMessageListIterator msgList_itr;
|
|
|
|
public:
|
|
FGATCDisplay();
|
|
~FGATCDisplay();
|
|
|
|
void init();
|
|
|
|
void bind();
|
|
|
|
void unbind();
|
|
|
|
// Display any registered messages
|
|
void update(double dt);
|
|
|
|
// Register a single message for display after a delay of delay seconds
|
|
// Will automatically stop displaying after a suitable interval.
|
|
void RegisterSingleMessage(string msg, int delay); // OK - I know passing a string in and out is probably not good but it will have to do for now.
|
|
|
|
// For now we will assume only one repeating message at once
|
|
// This is not really robust
|
|
|
|
// Register a continuously repeating message
|
|
void RegisterRepeatingMessage(string msg);
|
|
|
|
// Change a repeating message - assume that the message changes after the string has finished for now
|
|
void ChangeRepeatingMessage(string newmsg);
|
|
|
|
// Cancel the current repeating message
|
|
void CancelRepeatingMessage();
|
|
};
|
|
|
|
#endif // _FG_ATC_DISPLAY_HXX
|