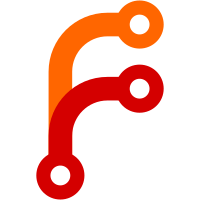
Switch to defining PU_USE_NONE and providing our own callback functions to pui for "get window" and "get window size." A new WindowSystemAdapter class assigns ID numbers to windows for the purpose of identifying them to plib; the window size can be extracted from the osg::GraphicsContext class in all the different implementations (osgViewer, glut, sdl). Implement a GraphicsContextOperation that runs code in a particular graphics context, perhaps in another thread, and provides an isFinished() method to test if the operation has finished. This allows us to initialize plib PUI properly if there are multiple graphics contexts without using fgMakeCurrent(). fgMakeCurrent() can't work in multi-threaded OSG configurations. Eliminate fgMakeCurrent() and all its uses, either by using GraphicsContextOperation or by seeing that it is not necessary. Attach the GUI camera as a slave camera. Don't manipulate the OSG state in the drawImplementation() functions for SGHUDAndPanelDrawable and SGPuDrawable; it's not needed.
108 lines
3 KiB
C
108 lines
3 KiB
C
/**************************************************************************
|
|
* gui.h
|
|
*
|
|
* Written 1998 by Durk Talsma, started Juni, 1998. For the flight gear
|
|
* project.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of the
|
|
* License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
*
|
|
* $Id$
|
|
**************************************************************************/
|
|
|
|
|
|
#ifndef _GUI_H_
|
|
#define _GUI_H_
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
# include <config.h>
|
|
#endif
|
|
|
|
#ifdef HAVE_WINDOWS_H
|
|
# include <windows.h>
|
|
#endif
|
|
|
|
#include <plib/pu.h>
|
|
|
|
#include <simgear/structure/exception.hxx>
|
|
|
|
#define TR_HIRES_SNAP 1
|
|
|
|
|
|
// gui.cxx
|
|
extern void guiStartInit();
|
|
extern bool guiFinishInit();
|
|
extern void mkDialog(const char *txt);
|
|
extern void guiErrorMessage(const char *txt);
|
|
extern void guiErrorMessage(const char *txt, const sg_throwable &throwable);
|
|
|
|
extern bool fgDumpSnapShot();
|
|
extern void fgDumpSceneGraph();
|
|
extern void fgDumpTerrainBranch();
|
|
|
|
extern puFont guiFnt;
|
|
extern fntTexFont *guiFntHandle;
|
|
extern int gui_menu_on;
|
|
|
|
// from gui_funcs.cxx
|
|
extern void reInit(puObject *);
|
|
extern void fgDumpSnapShotWrapper(puObject *);
|
|
#ifdef TR_HIRES_SNAP
|
|
extern void fgHiResDumpWrapper(puObject *);
|
|
extern void fgHiResDump();
|
|
#endif
|
|
#if defined( WIN32 ) && !defined( __CYGWIN__) && !defined(__MINGW32__)
|
|
extern void printScreen(puObject *);
|
|
#endif
|
|
extern void helpCb(puObject *);
|
|
|
|
typedef struct {
|
|
const char *name;
|
|
void (*fn)(puObject *);
|
|
} __fg_gui_fn_t;
|
|
extern const __fg_gui_fn_t __fg_gui_fn[];
|
|
|
|
// mouse.cxx
|
|
extern void guiInitMouse(int width, int height);
|
|
extern void maybeToggleMouse( void );
|
|
extern void TurnCursorOn( void );
|
|
extern void TurnCursorOff( void );
|
|
|
|
// MACROS TO HELP KEEP PUI LIVE INTERFACE STACK IN SYNC
|
|
// These insure that the mouse is active when dialog is shown
|
|
// and try to the maintain the original mouse state when hidden
|
|
// These will also repair any damage done to the Panel if active
|
|
|
|
// Activate Dialog Box
|
|
inline void FG_PUSH_PUI_DIALOG( puObject *X ) {
|
|
maybeToggleMouse();
|
|
puPushLiveInterface( (puInterface *)X ) ;
|
|
X->reveal() ;
|
|
}
|
|
|
|
// Deactivate Dialog Box
|
|
inline void FG_POP_PUI_DIALOG( puObject *X ) {
|
|
X->hide();
|
|
puPopLiveInterface();
|
|
maybeToggleMouse();
|
|
}
|
|
|
|
// Finalize Dialog Box Construction
|
|
inline void FG_FINALIZE_PUI_DIALOG( puObject *X ) {
|
|
((puGroup *)X)->close();
|
|
X->hide();
|
|
puPopLiveInterface();
|
|
}
|
|
|
|
#endif // _GUI_H_
|