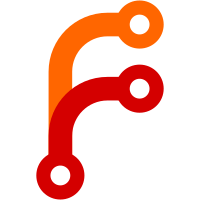
and "isOnRunway". - Added initial support for AI controlled pushback operations, making use of the current editing capabilities of TaxiDraw CVS / New_GUI_CODE. The current implementation is slightly more computationally intensive than strictly required, due to the currently inability of taxidraw to link one specific pushBack point to to a particular startup location. FlightGear now determines this dynamically, and once we have that functionality in TaxiDraw, the initialization part of createPushBack() can be further simplified. - Smoother transition from pushback to taxi. No more skipping of waypoints, and aircraft wait for two minutes at pushback point. - The classes FGTaxiNode, FGTaxiSegment, and FGParking, now have copy constructors, and assignment operators. - Removed declaration of undefined constructor FGTaxiNode(double, double, int) - Array boundry checks and cleanup. - Modified Dijkstra path search algoritm to solve partial problems. Currently limited to include pushback points and routes only, but can probably be extended to a more general approach. - Added initial support for giving certain routes in the network a penalty, in order to discourage the use of certain routes over others.
53 lines
1.4 KiB
C++
53 lines
1.4 KiB
C++
#include "gnnode.hxx"
|
|
#include "groundnetwork.hxx"
|
|
|
|
#include <algorithm>
|
|
SG_USING_STD(sort);
|
|
|
|
/*****************************************************************************
|
|
* Helper function for parsing position string
|
|
****************************************************************************/
|
|
double processPosition(const string &pos)
|
|
{
|
|
string prefix;
|
|
string subs;
|
|
string degree;
|
|
string decimal;
|
|
int sign = 1;
|
|
double value;
|
|
subs = pos;
|
|
prefix= subs.substr(0,1);
|
|
if (prefix == string("S") || (prefix == string("W")))
|
|
sign = -1;
|
|
subs = subs.substr(1, subs.length());
|
|
degree = subs.substr(0, subs.find(" ",0));
|
|
decimal = subs.substr(subs.find(" ",0), subs.length());
|
|
|
|
|
|
//cerr << sign << " "<< degree << " " << decimal << endl;
|
|
value = sign * (atof(degree.c_str()) + atof(decimal.c_str())/60.0);
|
|
//cerr << value <<endl;
|
|
//exit(1);
|
|
return value;
|
|
}
|
|
|
|
bool sortByHeadingDiff(FGTaxiSegment *a, FGTaxiSegment *b) {
|
|
return a->hasSmallerHeadingDiff(*b);
|
|
}
|
|
|
|
bool sortByLength(FGTaxiSegment *a, FGTaxiSegment *b) {
|
|
return a->getLength() > b->getLength();
|
|
}
|
|
|
|
/**************************************************************************
|
|
* FGTaxiNode
|
|
*************************************************************************/
|
|
|
|
|
|
void FGTaxiNode::sortEndSegments(bool byLength)
|
|
{
|
|
if (byLength)
|
|
sort(next.begin(), next.end(), sortByLength);
|
|
else
|
|
sort(next.begin(), next.end(), sortByHeadingDiff);
|
|
}
|