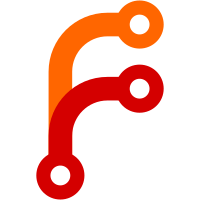
(i.e. multiloop). Most subsystems currently ignore the parameter, but eventually, it will allow all subsystems to update by time rather than by framerate.
105 lines
3.4 KiB
C++
105 lines
3.4 KiB
C++
// MagicCarpet.cxx -- interface to the "Magic Carpet" flight model
|
|
//
|
|
// Written by Curtis Olson, started October 1999.
|
|
//
|
|
// Copyright (C) 1999 Curtis L. Olson - curt@flightgear.org
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of the GNU General Public License as
|
|
// published by the Free Software Foundation; either version 2 of the
|
|
// License, or (at your option) any later version.
|
|
//
|
|
// This program is distributed in the hope that it will be useful, but
|
|
// WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU General Public License
|
|
// along with this program; if not, write to the Free Software
|
|
// Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
//
|
|
// $Id$
|
|
|
|
|
|
#include <simgear/math/sg_geodesy.hxx>
|
|
#include <simgear/math/point3d.hxx>
|
|
#include <simgear/math/polar3d.hxx>
|
|
|
|
#include <Controls/controls.hxx>
|
|
#include <Main/globals.hxx>
|
|
#include <Main/fg_props.hxx>
|
|
|
|
#include "MagicCarpet.hxx"
|
|
|
|
|
|
FGMagicCarpet::FGMagicCarpet( double dt ) {
|
|
set_delta_t( dt );
|
|
}
|
|
|
|
|
|
FGMagicCarpet::~FGMagicCarpet() {
|
|
}
|
|
|
|
|
|
// Initialize the Magic Carpet flight model, dt is the time increment
|
|
// for each subsequent iteration through the EOM
|
|
void FGMagicCarpet::init() {
|
|
common_init();
|
|
}
|
|
|
|
|
|
// Run an iteration of the EOM (equations of motion)
|
|
void FGMagicCarpet::update( int multiloop ) {
|
|
// cout << "FGLaRCsim::update()" << endl;
|
|
|
|
double time_step = get_delta_t() * multiloop;
|
|
|
|
// speed and distance traveled
|
|
double speed = globals->get_controls()->get_throttle( 0 ) * 2000; // meters/sec
|
|
double dist = speed * time_step;
|
|
double kts = speed * SG_METER_TO_NM * 3600.0;
|
|
_set_V_equiv_kts( kts );
|
|
_set_V_calibrated_kts( kts );
|
|
_set_V_ground_speed( kts );
|
|
|
|
// angle of turn
|
|
double turn_rate = globals->get_controls()->get_aileron() * SGD_PI_4; // radians/sec
|
|
double turn = turn_rate * time_step;
|
|
|
|
// update euler angles
|
|
_set_Euler_Angles( get_Phi(), get_Theta(),
|
|
fmod(get_Psi() + turn, SGD_2PI) );
|
|
_set_Euler_Rates(0,0,0);
|
|
|
|
// update (lon/lat) position
|
|
double lat2, lon2, az2;
|
|
if ( speed > SG_EPSILON ) {
|
|
geo_direct_wgs_84 ( get_Altitude(),
|
|
get_Latitude() * SGD_RADIANS_TO_DEGREES,
|
|
get_Longitude() * SGD_RADIANS_TO_DEGREES,
|
|
get_Psi() * SGD_RADIANS_TO_DEGREES,
|
|
dist, &lat2, &lon2, &az2 );
|
|
|
|
_set_Longitude( lon2 * SGD_DEGREES_TO_RADIANS );
|
|
_set_Latitude( lat2 * SGD_DEGREES_TO_RADIANS );
|
|
}
|
|
|
|
// cout << "lon error = " << fabs(end.x()*SGD_RADIANS_TO_DEGREES - lon2)
|
|
// << " lat error = " << fabs(end.y()*SGD_RADIANS_TO_DEGREES - lat2)
|
|
// << endl;
|
|
|
|
double sl_radius, lat_geoc;
|
|
sgGeodToGeoc( get_Latitude(), get_Altitude(), &sl_radius, &lat_geoc );
|
|
|
|
// update altitude
|
|
double real_climb_rate = -globals->get_controls()->get_elevator() * 5000; // feet/sec
|
|
_set_Climb_Rate( real_climb_rate / 500.0 );
|
|
double climb = real_climb_rate * time_step;
|
|
|
|
_set_Geocentric_Position( lat_geoc, get_Longitude(),
|
|
sl_radius + get_Altitude() + climb );
|
|
// cout << "sea level radius (ft) = " << sl_radius << endl;
|
|
// cout << "(setto) sea level radius (ft) = " << get_Sea_level_radius() << endl;
|
|
_set_Sea_level_radius( sl_radius * SG_METER_TO_FEET);
|
|
_set_Altitude( get_Altitude() + climb );
|
|
}
|