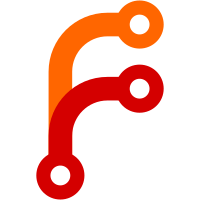
This is a new improved patch for the previous tile manager fixes. Rather than building dependencies between FGlocation or the viewer or fdm with tilemgr what I ended up doing was linking the pieces together in the Mainloop in main.cxx. You'll see what I mean...it's been commented fairly well. More than likely we should move that chunk somewhere...just not sure where yet. The changes seem clean now. As I get more ideas there could be some further improvement in organizing the update in tilemgr. You'll note that I left an override in there for the tilemgr::update() function to preserve earlier functionality if someone needs it (e.g. usage independent of an fdm or viewer), not to mention there are a few places in flightgear that call it directly that have not been changed to the new interface (and may not need to be). The code has been optimized to avoid duplicate traversals and seems to run generally quite well. Note that there can be a short delay reloading tiles that have been dropped from static views. We could call the tile scheduler on a view switch, but it's not a big deal and at the moment I'd like to get this in so people can try it and comment on it as it is. Everything has been resycned with CVS tonight and I've included the description submitted earlier (below). Best, Jim Changes synced with CVS approx 20:30EDT 2002-05-09 (after this evenings updates). Files: http://www.spiderbark.com/fgfs/viewer-update-20020516.tar.gz or http://www.spiderbark.com/fgfs/viewer-update-20020516.diffs.gz Description: In a nutshell, these patches begin to take what was one value for ground elevation and calculate ground elevation values seperately for the FDM and the viewer (eye position). Several outstanding view related bugs have been fixed. With the introduction of the new viewer code a lot of that Flight Gear code broke related to use of a global variable called "scenery.cur_elev". Therefore the ground_elevation and other associated items (like the current tile bucket) is maintained per FDM instance and per View. Each of these has a "point" or location that can be identified. See changes to FGLocation class and main.cxx. Most of the problems related to the new viewer in terms of sky, ground and runway lights, and tower views are fixed. There are four minor problems remaining. 1) The sun/moon spins when you pan the "lookat" tower view only (view #3). 2) Under stress (esp. magic carpet full speed with max visibility), there is a memory leak in the tile caching that was not introduced with these changes. 3) I have not tested these changes or made corrections to the ADA or External FDM interfaces. 4) The change view function doesn't call the time/light update (not a problem unless a tower is very far away). Details: FDM/flight.cxx, flight.hxx - FGInterface ties to FGAircraftModel so that it's location data can be accessed for runway (ground elevation under aircraft) elevation. FDM/larsim.cxx, larcsim.hxx - gets runway elevation from FGInterface now. Commented out function that is causing a namespace conflict, hasn't been called with recent code anyway. FDM/JSBSim/JSBSim.cxx, YASim/YASim.cxx - gets runway elevation from FGInterface now. Scenery/newcache.cxx, newcache.hxx - changed caching scheme to time based (oldest tiles discard). Scenery/tileentry.cxx, tileentry.hxx - added place to record time, changed rendering to reference viewer altitude in order to fix a problem with ground and runway lights. Scenery/tilemgr.cxx, tilemgr.hxx - Modified update() to accept values for multiple locations. Refresh function added in order to periodically make the tiles current for a non-moving view (like a tower). Main/fg_init.cxx - register event for making tiles current in a non-moving view (like a tower). Main/location.hxx - added support for current ground elevation data. Main/main.cxx - added second tilemgr call for fdm, fixed places where viewer position data was required for correct sky rendering. Main/options.cxx - fixed segfault reported by Curtis when using --view-offset command line parameter. Main/viewer.cxx, viewer.hxx - removed fudging of view position. Fixed numerous bugs that were causing eye and target values to get mixed up.
200 lines
5.3 KiB
C++
200 lines
5.3 KiB
C++
// tilemgr.hxx -- routines to handle dynamic management of scenery tiles
|
|
//
|
|
// Written by Curtis Olson, started January 1998.
|
|
//
|
|
// Copyright (C) 1997 Curtis L. Olson - curt@infoplane.com
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of the GNU General Public License as
|
|
// published by the Free Software Foundation; either version 2 of the
|
|
// License, or (at your option) any later version.
|
|
//
|
|
// This program is distributed in the hope that it will be useful, but
|
|
// WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU General Public License
|
|
// along with this program; if not, write to the Free Software
|
|
// Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
//
|
|
// $Id$
|
|
|
|
|
|
#ifndef _TILEMGR_HXX
|
|
#define _TILEMGR_HXX
|
|
|
|
|
|
#ifndef __cplusplus
|
|
# error This library requires C++
|
|
#endif
|
|
|
|
#include <simgear/compiler.h>
|
|
|
|
#include <queue>
|
|
|
|
#include <plib/ssg.h>
|
|
|
|
#include <simgear/bucket/newbucket.hxx>
|
|
#ifdef ENABLE_THREADS
|
|
# include <simgear/threads/SGQueue.hxx>
|
|
#endif // ENABLE_THREADS
|
|
|
|
#include "FGTileLoader.hxx"
|
|
#include "hitlist.hxx"
|
|
#include "newcache.hxx"
|
|
|
|
#if defined(USE_MEM) || defined(WIN32)
|
|
# define FG_MEM_COPY(to,from,n) memcpy(to, from, n)
|
|
#else
|
|
# define FG_MEM_COPY(to,from,n) bcopy(from, to, n)
|
|
#endif
|
|
|
|
SG_USING_STD( queue );
|
|
|
|
|
|
// forward declaration
|
|
class FGTileEntry;
|
|
class FGDeferredModel;
|
|
|
|
|
|
class FGTileMgr {
|
|
|
|
private:
|
|
|
|
// Tile loading state
|
|
enum load_state {
|
|
Start = 0,
|
|
Inited = 1,
|
|
Running = 2
|
|
};
|
|
|
|
load_state state;
|
|
|
|
// initialize the cache
|
|
void initialize_queue();
|
|
|
|
// schedule a tile for loading
|
|
void sched_tile( const SGBucket& b );
|
|
|
|
// schedule a needed buckets for loading
|
|
void schedule_needed(double visibility_meters, SGBucket curr_bucket);
|
|
|
|
// see comment at prep_ssg_nodes()
|
|
void prep_ssg_node( int idx );
|
|
|
|
FGHitList hit_list;
|
|
|
|
SGBucket previous_bucket;
|
|
SGBucket current_bucket;
|
|
SGBucket pending;
|
|
|
|
FGTileEntry *current_tile;
|
|
|
|
// x and y distance of tiles to load/draw
|
|
float vis;
|
|
int xrange, yrange;
|
|
|
|
// current longitude latitude
|
|
double longitude;
|
|
double latitude;
|
|
double last_longitude;
|
|
double last_latitude;
|
|
|
|
/**
|
|
* tile cache
|
|
*/
|
|
FGNewCache tile_cache;
|
|
|
|
/**
|
|
* Queue tiles for loading.
|
|
*/
|
|
FGTileLoader loader;
|
|
int counter_hack;
|
|
|
|
/**
|
|
* Work queues.
|
|
*
|
|
* attach_queue is the tiles that have been loaded [by the pager]
|
|
* that can be attached to the scene graph by the render thread.
|
|
*
|
|
* model_queue is the set of models that need to be loaded by the
|
|
* primary render thread.
|
|
*/
|
|
#ifdef ENABLE_THREADS
|
|
static SGLockedQueue<FGTileEntry *> attach_queue;
|
|
static SGLockedQueue<FGDeferredModel *> model_queue;
|
|
#else
|
|
static queue<FGTileEntry *> attach_queue;
|
|
static queue<FGDeferredModel *> model_queue;
|
|
#endif // ENABLE_THREADS
|
|
|
|
public:
|
|
|
|
/**
|
|
* Add a loaded tile to the 'attach to the scene graph' queue.
|
|
*/
|
|
static void ready_to_attach( FGTileEntry *t ) { attach_queue.push( t ); }
|
|
|
|
#ifdef WISH_PLIB_WAS_THREADED // but it isn't
|
|
/**
|
|
* Tile is detatched from scene graph and is ready to delete
|
|
*/
|
|
inline void ready_to_delete( FGTileEntry *t ) { loader.remove( t ); }
|
|
#endif
|
|
|
|
/**
|
|
* Add a pending model to the 'deferred model load' queue
|
|
*/
|
|
static void model_ready( FGDeferredModel *dm ) { model_queue.push( dm ); }
|
|
|
|
public:
|
|
|
|
// Constructor
|
|
FGTileMgr();
|
|
|
|
// Destructor
|
|
~FGTileMgr();
|
|
|
|
// Initialize the Tile Manager subsystem
|
|
int init();
|
|
|
|
// given the current lon/lat (in degrees), fill in the array of
|
|
// local chunks. If the chunk isn't already in the cache, then
|
|
// read it from disk.
|
|
int update( double lon, double lat, double visibility_meters );
|
|
int update( double lon, double lat, double visibility_meters, sgdVec3 abs_pos_vector, SGBucket p_current, SGBucket p_previous );
|
|
void setCurrentTile( double longitude, double latitude );
|
|
void updateCurrentElevAtPos(sgdVec3 abs_pos_vector);
|
|
|
|
// Determine scenery altitude. Normally this just happens when we
|
|
// render the scene, but we'd also like to be able to do this
|
|
// explicitely. lat & lon are in radians. abs_view_pos in
|
|
// meters. Returns result in meters.
|
|
void my_ssg_los( string s, ssgBranch *branch, sgdMat4 m,
|
|
const sgdVec3 p, const sgdVec3 dir, sgdVec3 normal );
|
|
|
|
void my_ssg_los( ssgBranch *branch, sgdMat4 m,
|
|
const sgdVec3 p, const sgdVec3 dir,
|
|
FGHitList *list );
|
|
|
|
// Prepare the ssg nodes ... for each tile, set it's proper
|
|
// transform and update it's range selector based on current
|
|
// visibilty
|
|
void prep_ssg_nodes(float visibility_meters);
|
|
|
|
//
|
|
// Set flag with event manager so that non-moving view refreshes tiles...
|
|
//
|
|
void refresh_view_timestamps();
|
|
|
|
inline SGBucket get_current_bucket () { return current_bucket; }
|
|
inline SGBucket get_previous_bucket () { return previous_bucket; }
|
|
};
|
|
|
|
|
|
// the tile manager
|
|
extern FGTileMgr global_tile_mgr;
|
|
|
|
|
|
#endif // _TILEMGR_HXX
|