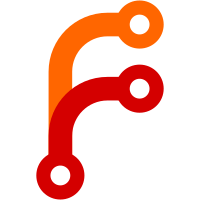
This is a new improved patch for the previous tile manager fixes. Rather than building dependencies between FGlocation or the viewer or fdm with tilemgr what I ended up doing was linking the pieces together in the Mainloop in main.cxx. You'll see what I mean...it's been commented fairly well. More than likely we should move that chunk somewhere...just not sure where yet. The changes seem clean now. As I get more ideas there could be some further improvement in organizing the update in tilemgr. You'll note that I left an override in there for the tilemgr::update() function to preserve earlier functionality if someone needs it (e.g. usage independent of an fdm or viewer), not to mention there are a few places in flightgear that call it directly that have not been changed to the new interface (and may not need to be). The code has been optimized to avoid duplicate traversals and seems to run generally quite well. Note that there can be a short delay reloading tiles that have been dropped from static views. We could call the tile scheduler on a view switch, but it's not a big deal and at the moment I'd like to get this in so people can try it and comment on it as it is. Everything has been resycned with CVS tonight and I've included the description submitted earlier (below). Best, Jim Changes synced with CVS approx 20:30EDT 2002-05-09 (after this evenings updates). Files: http://www.spiderbark.com/fgfs/viewer-update-20020516.tar.gz or http://www.spiderbark.com/fgfs/viewer-update-20020516.diffs.gz Description: In a nutshell, these patches begin to take what was one value for ground elevation and calculate ground elevation values seperately for the FDM and the viewer (eye position). Several outstanding view related bugs have been fixed. With the introduction of the new viewer code a lot of that Flight Gear code broke related to use of a global variable called "scenery.cur_elev". Therefore the ground_elevation and other associated items (like the current tile bucket) is maintained per FDM instance and per View. Each of these has a "point" or location that can be identified. See changes to FGLocation class and main.cxx. Most of the problems related to the new viewer in terms of sky, ground and runway lights, and tower views are fixed. There are four minor problems remaining. 1) The sun/moon spins when you pan the "lookat" tower view only (view #3). 2) Under stress (esp. magic carpet full speed with max visibility), there is a memory leak in the tile caching that was not introduced with these changes. 3) I have not tested these changes or made corrections to the ADA or External FDM interfaces. 4) The change view function doesn't call the time/light update (not a problem unless a tower is very far away). Details: FDM/flight.cxx, flight.hxx - FGInterface ties to FGAircraftModel so that it's location data can be accessed for runway (ground elevation under aircraft) elevation. FDM/larsim.cxx, larcsim.hxx - gets runway elevation from FGInterface now. Commented out function that is causing a namespace conflict, hasn't been called with recent code anyway. FDM/JSBSim/JSBSim.cxx, YASim/YASim.cxx - gets runway elevation from FGInterface now. Scenery/newcache.cxx, newcache.hxx - changed caching scheme to time based (oldest tiles discard). Scenery/tileentry.cxx, tileentry.hxx - added place to record time, changed rendering to reference viewer altitude in order to fix a problem with ground and runway lights. Scenery/tilemgr.cxx, tilemgr.hxx - Modified update() to accept values for multiple locations. Refresh function added in order to periodically make the tiles current for a non-moving view (like a tower). Main/fg_init.cxx - register event for making tiles current in a non-moving view (like a tower). Main/location.hxx - added support for current ground elevation data. Main/main.cxx - added second tilemgr call for fdm, fixed places where viewer position data was required for correct sky rendering. Main/options.cxx - fixed segfault reported by Curtis when using --view-offset command line parameter. Main/viewer.cxx, viewer.hxx - removed fudging of view position. Fixed numerous bugs that were causing eye and target values to get mixed up.
293 lines
8.4 KiB
C++
293 lines
8.4 KiB
C++
// newcache.cxx -- routines to handle scenery tile caching
|
|
//
|
|
// Written by Curtis Olson, started December 2000.
|
|
//
|
|
// Copyright (C) 2000 Curtis L. Olson - curt@flightgear.org
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of the GNU General Public License as
|
|
// published by the Free Software Foundation; either version 2 of the
|
|
// License, or (at your option) any later version.
|
|
//
|
|
// This program is distributed in the hope that it will be useful, but
|
|
// WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU General Public License
|
|
// along with this program; if not, write to the Free Software
|
|
// Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
//
|
|
// $Id$
|
|
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
# include <config.h>
|
|
#endif
|
|
|
|
#ifdef HAVE_WINDOWS_H
|
|
# include <windows.h>
|
|
#endif
|
|
|
|
#include <GL/glut.h>
|
|
#include <GL/gl.h>
|
|
|
|
#include <plib/ssg.h> // plib include
|
|
|
|
#include <simgear/bucket/newbucket.hxx>
|
|
#include <simgear/debug/logstream.hxx>
|
|
#include <simgear/misc/sg_path.hxx>
|
|
|
|
#include <Main/globals.hxx>
|
|
#include <Main/viewer.hxx>
|
|
#include <Scenery/scenery.hxx> // for scenery.center
|
|
|
|
#include "newcache.hxx"
|
|
#include "tileentry.hxx"
|
|
|
|
|
|
SG_USING_NAMESPACE(std);
|
|
|
|
|
|
// Constructor
|
|
FGNewCache::FGNewCache( void ) :
|
|
max_cache_size(100)
|
|
{
|
|
tile_cache.clear();
|
|
}
|
|
|
|
|
|
// Destructor
|
|
FGNewCache::~FGNewCache( void ) {
|
|
}
|
|
|
|
|
|
// Free a tile cache entry
|
|
void FGNewCache::entry_free( long cache_index ) {
|
|
SG_LOG( SG_TERRAIN, SG_DEBUG, "FREEING CACHE ENTRY = " << cache_index );
|
|
FGTileEntry *tile = tile_cache[cache_index];
|
|
tile->disconnect_ssg_nodes();
|
|
|
|
#ifdef WISH_PLIB_WAS_THREADED // but it isn't
|
|
tile->sched_removal();
|
|
#else
|
|
tile->free_tile();
|
|
delete tile;
|
|
#endif
|
|
|
|
tile_cache.erase( cache_index );
|
|
}
|
|
|
|
|
|
// Initialize the tile cache subsystem
|
|
void FGNewCache::init( void ) {
|
|
SG_LOG( SG_TERRAIN, SG_INFO, "Initializing the tile cache." );
|
|
|
|
SG_LOG( SG_TERRAIN, SG_INFO, " max cache size = "
|
|
<< max_cache_size );
|
|
SG_LOG( SG_TERRAIN, SG_INFO, " current cache size = "
|
|
<< tile_cache.size() );
|
|
|
|
#if 0 // don't clear the cache
|
|
clear_cache();
|
|
#endif
|
|
|
|
SG_LOG( SG_TERRAIN, SG_INFO, " done with init()" );
|
|
}
|
|
|
|
|
|
// Search for the specified "bucket" in the cache
|
|
bool FGNewCache::exists( const SGBucket& b ) const {
|
|
long tile_index = b.gen_index();
|
|
const_tile_map_iterator it = tile_cache.find( tile_index );
|
|
|
|
return ( it != tile_cache.end() );
|
|
}
|
|
|
|
|
|
// Ensure at least one entry is free in the cache
|
|
bool FGNewCache::make_space() {
|
|
SG_LOG( SG_TERRAIN, SG_DEBUG, "Make space in cache" );
|
|
SG_LOG( SG_TERRAIN, SG_DEBUG, "cache entries = " << tile_cache.size() );
|
|
SG_LOG( SG_TERRAIN, SG_DEBUG, "max size = " << max_cache_size );
|
|
|
|
if ( (int)tile_cache.size() < max_cache_size ) {
|
|
// space in the cache, return
|
|
return true;
|
|
}
|
|
|
|
while ( (int)tile_cache.size() >= max_cache_size ) {
|
|
sgdVec3 abs_view_pos;
|
|
float dist;
|
|
double timestamp = 0.0;
|
|
int max_index = -1;
|
|
double min_time = 2419200000.0f; // one month should be enough
|
|
double max_time = 0;
|
|
|
|
// we need to free the furthest entry
|
|
tile_map_iterator current = tile_cache.begin();
|
|
tile_map_iterator end = tile_cache.end();
|
|
|
|
for ( ; current != end; ++current ) {
|
|
long index = current->first;
|
|
FGTileEntry *e = current->second;
|
|
if ( e->is_loaded() && (e->get_pending_models() == 0) ) {
|
|
|
|
timestamp = e->get_timestamp();
|
|
if ( timestamp < min_time ) {
|
|
max_index = index;
|
|
min_time = timestamp;
|
|
}
|
|
if ( timestamp > max_time ) {
|
|
max_time = timestamp;
|
|
}
|
|
|
|
} else {
|
|
SG_LOG( SG_TERRAIN, SG_INFO, "loaded = " << e->is_loaded()
|
|
<< " pending models = " << e->get_pending_models()
|
|
<< " time stamp = " << e->get_timestamp() );
|
|
}
|
|
}
|
|
|
|
// If we made it this far, then there were no open cache entries.
|
|
// We will instead free the oldest cache entry and return true
|
|
|
|
SG_LOG( SG_TERRAIN, SG_INFO, " min_time = " << min_time );
|
|
SG_LOG( SG_TERRAIN, SG_INFO, " index = " << max_index );
|
|
SG_LOG( SG_TERRAIN, SG_INFO, " max_time = " << max_time );
|
|
if ( max_index >= 0 ) {
|
|
entry_free( max_index );
|
|
return true;
|
|
} else {
|
|
SG_LOG( SG_TERRAIN, SG_ALERT, "WHOOPS!!! can't make_space(), tile "
|
|
"cache is full, but no entries available for removal." );
|
|
return false;
|
|
}
|
|
}
|
|
|
|
SG_LOG( SG_TERRAIN, SG_ALERT, "WHOOPS!!! Hit an unhandled condition in "
|
|
"FGNewCache::make_space()." );
|
|
return false;
|
|
}
|
|
|
|
|
|
// Clear all completely loaded tiles (ignores partially loaded tiles)
|
|
void FGNewCache::clear_cache() {
|
|
|
|
tile_map_iterator current = tile_cache.begin();
|
|
tile_map_iterator end = tile_cache.end();
|
|
|
|
for ( ; current != end; ++current ) {
|
|
long index = current->first;
|
|
SG_LOG( SG_TERRAIN, SG_DEBUG, "clearing " << index );
|
|
FGTileEntry *e = current->second;
|
|
if ( e->is_loaded() && (e->get_pending_models() == 0) ) {
|
|
e->tile_bucket.make_bad();
|
|
entry_free(index);
|
|
}
|
|
}
|
|
|
|
// and ... just in case we missed something ...
|
|
globals->get_scenery()->get_terrain_branch()->removeAllKids();
|
|
}
|
|
|
|
|
|
/**
|
|
* Create a new tile and schedule it for loading.
|
|
*/
|
|
bool FGNewCache::insert_tile( FGTileEntry *e ) {
|
|
// set time of insertion for tracking age of tiles...
|
|
e->set_timestamp(globals->get_sim_time_sec());
|
|
// clear out a distant entry in the cache if needed.
|
|
if ( make_space() ) {
|
|
// register it in the cache
|
|
long tile_index = e->get_tile_bucket().gen_index();
|
|
tile_cache[tile_index] = e;
|
|
|
|
return true;
|
|
} else {
|
|
// failed to find cache space
|
|
|
|
return false;
|
|
}
|
|
}
|
|
|
|
// Note this is the old version of FGNewCache::make_space(), currently disabled
|
|
// It uses distance from a center point to determine tiles to be discarded...
|
|
#ifdef 0
|
|
// Ensure at least one entry is free in the cache
|
|
bool FGNewCache::make_space() {
|
|
SG_LOG( SG_TERRAIN, SG_DEBUG, "Make space in cache" );
|
|
SG_LOG( SG_TERRAIN, SG_DEBUG, "cache entries = " << tile_cache.size() );
|
|
SG_LOG( SG_TERRAIN, SG_DEBUG, "max size = " << max_cache_size );
|
|
|
|
if ( (int)tile_cache.size() < max_cache_size ) {
|
|
// space in the cache, return
|
|
return true;
|
|
}
|
|
|
|
while ( (int)tile_cache.size() >= max_cache_size ) {
|
|
sgdVec3 abs_view_pos;
|
|
float dist;
|
|
float max_dist = 0.0;
|
|
int max_index = -1;
|
|
|
|
// we need to free the furthest entry
|
|
tile_map_iterator current = tile_cache.begin();
|
|
tile_map_iterator end = tile_cache.end();
|
|
|
|
for ( ; current != end; ++current ) {
|
|
long index = current->first;
|
|
FGTileEntry *e = current->second;
|
|
|
|
if ( e->is_loaded() && (e->get_pending_models() == 0) ) {
|
|
// calculate approximate distance from view point
|
|
sgdCopyVec3( abs_view_pos,
|
|
globals->get_current_view()->get_absolute_view_pos() );
|
|
|
|
SG_LOG( SG_TERRAIN, SG_DEBUG, "DIST Abs view pos = "
|
|
<< abs_view_pos[0] << ","
|
|
<< abs_view_pos[1] << ","
|
|
<< abs_view_pos[2] );
|
|
SG_LOG( SG_TERRAIN, SG_DEBUG,
|
|
" ref point = " << e->center );
|
|
|
|
sgdVec3 center;
|
|
sgdSetVec3( center,
|
|
e->center.x(), e->center.y(), e->center.z() );
|
|
dist = sgdDistanceVec3( center, abs_view_pos );
|
|
|
|
SG_LOG( SG_TERRAIN, SG_DEBUG, " distance = " << dist );
|
|
|
|
if ( dist > max_dist ) {
|
|
max_dist = dist;
|
|
max_index = index;
|
|
}
|
|
} else {
|
|
SG_LOG( SG_TERRAIN, SG_INFO, "loaded = " << e->is_loaded()
|
|
<< " pending models = " << e->get_pending_models() );
|
|
}
|
|
}
|
|
|
|
// If we made it this far, then there were no open cache entries.
|
|
// We will instead free the furthest cache entry and return true
|
|
|
|
SG_LOG( SG_TERRAIN, SG_INFO, " max_dist = " << max_dist );
|
|
SG_LOG( SG_TERRAIN, SG_INFO, " index = " << max_index );
|
|
if ( max_index >= 0 ) {
|
|
entry_free( max_index );
|
|
return true;
|
|
} else {
|
|
SG_LOG( SG_TERRAIN, SG_ALERT, "WHOOPS!!! can't make_space(), tile "
|
|
"cache is full, but no entries available for removal." );
|
|
return false;
|
|
}
|
|
}
|
|
|
|
SG_LOG( SG_TERRAIN, SG_ALERT, "WHOOPS!!! Hit an unhandled condition in "
|
|
"FGNewCache::make_space()." );
|
|
return false;
|
|
}
|
|
#endif
|
|
|
|
|