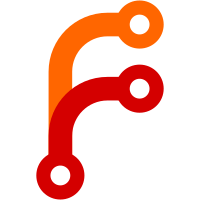
Syd Adams: I've added an update to dme.cxx /dme.hxx. It adds a formatted KDI572-574/nm , min and kt string properties meant for text animations for these instruments.Existing instruments will funtion as usual, no breaks. The purpose here is to eliminate yet another nasal workaround which usually needs to repeat some of what the code already does. The 3 properties are empty when the dme is off (no need to check for power), dashes when powered up but no source , out of range ,etc. The are formatted to the KDI-572/573/574 limits ... 0-99.9/100-389 for distance to station, 0-99 minutes for time to station, groundspeed 0-999 kt.
80 lines
1.8 KiB
C++
80 lines
1.8 KiB
C++
// dme.hxx - distance-measuring equipment.
|
|
// Written by David Megginson, started 2003.
|
|
//
|
|
// This file is in the Public Domain and comes with no warranty.
|
|
|
|
|
|
#ifndef __INSTRUMENTS_DME_HXX
|
|
#define __INSTRUMENTS_DME_HXX 1
|
|
|
|
#include <simgear/props/props.hxx>
|
|
#include <simgear/structure/subsystem_mgr.hxx>
|
|
|
|
// forward decls
|
|
class FGNavRecord;
|
|
|
|
/**
|
|
* Model a DME radio.
|
|
*
|
|
* Input properties:
|
|
*
|
|
* /position/longitude-deg
|
|
* /position/latitude-deg
|
|
* /position/altitude-ft
|
|
* /systems/electrical/outputs/dme
|
|
* /instrumentation/"name"/serviceable
|
|
* /instrumentation/"name"/frequencies/source
|
|
* /instrumentation/"name"/frequencies/selected-mhz
|
|
*
|
|
* Output properties:
|
|
*
|
|
* /instrumentation/"name"/in-range
|
|
* /instrumentation/"name"/indicated-distance-nm
|
|
* /instrumentation/"name"/indicated-ground-speed-kt
|
|
* /instrumentation/"name"/indicated-time-kt
|
|
*/
|
|
class DME : public SGSubsystem
|
|
{
|
|
|
|
public:
|
|
|
|
DME ( SGPropertyNode *node );
|
|
virtual ~DME ();
|
|
|
|
virtual void init ();
|
|
virtual void reinit ();
|
|
virtual void update (double delta_time_sec);
|
|
|
|
private:
|
|
|
|
SGPropertyNode_ptr _serviceable_node;
|
|
SGPropertyNode_ptr _electrical_node;
|
|
SGPropertyNode_ptr _source_node;
|
|
SGPropertyNode_ptr _frequency_node;
|
|
|
|
SGPropertyNode_ptr _in_range_node;
|
|
SGPropertyNode_ptr _distance_node;
|
|
SGPropertyNode_ptr _speed_node;
|
|
SGPropertyNode_ptr _time_node;
|
|
SGPropertyNode_ptr _ident_btn_node;
|
|
SGPropertyNode_ptr _volume_node;
|
|
|
|
SGPropertyNode_ptr _distance_string;
|
|
SGPropertyNode_ptr _speed_string;
|
|
SGPropertyNode_ptr _time_string;
|
|
|
|
double _last_distance_nm;
|
|
double _last_frequency_mhz;
|
|
double _time_before_search_sec;
|
|
|
|
FGNavRecord * _navrecord;
|
|
|
|
std::string _name;
|
|
|
|
int _num;
|
|
|
|
class AudioIdent * _audioIdent;
|
|
};
|
|
|
|
|
|
#endif // __INSTRUMENTS_DME_HXX
|