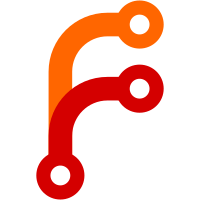
Remove leftover headers from plib/sg. Modified Files: src/AIModel/AIBase.cxx src/AIModel/AIFlightPlanCreateCruise.cxx src/ATCDCL/AIEntity.cxx src/ATCDCL/AIEntity.hxx src/ATCDCL/AIGAVFRTraffic.cxx src/ATCDCL/AIGAVFRTraffic.hxx src/ATCDCL/AILocalTraffic.cxx src/ATCDCL/AILocalTraffic.hxx src/ATCDCL/AIMgr.cxx src/ATCDCL/ATC.hxx src/ATCDCL/ATCDialog.cxx src/ATCDCL/ATCProjection.cxx src/ATCDCL/ATCProjection.hxx src/ATCDCL/ATCutils.cxx src/ATCDCL/ATCutils.hxx src/ATCDCL/approach.cxx src/ATCDCL/commlist.cxx src/ATCDCL/ground.cxx src/ATCDCL/ground.hxx src/ATCDCL/tower.cxx src/ATCDCL/tower.hxx src/Airports/calc_loc.cxx src/Airports/dynamics.cxx src/Airports/groundnetwork.cxx src/Airports/parking.cxx src/Airports/runwayprefs.cxx src/Airports/simple.cxx src/Cockpit/cockpit.cxx src/Cockpit/hud.hxx src/Cockpit/hud_card.cxx src/Cockpit/hud_rwy.cxx src/Environment/environment.cxx src/FDM/UFO.cxx src/FDM/SP/MagicCarpet.cxx src/GUI/dialog.hxx src/Instrumentation/HUD/HUD.hxx src/Instrumentation/HUD/HUD_runway.cxx src/Instrumentation/KLN89/kln89.cxx src/Main/fg_init.cxx src/Main/viewer.cxx src/Main/viewmgr.cxx src/Model/panelnode.cxx src/MultiPlayer/mpmessages.hxx src/Scenery/tilemgr.cxx src/Traffic/SchedFlight.cxx src/Traffic/TrafficMgr.cxx
71 lines
2.2 KiB
C++
71 lines
2.2 KiB
C++
// FGAIEntity - abstract base class an artificial intelligence entity
|
|
//
|
|
// Written by David Luff, started March 2002.
|
|
//
|
|
// Copyright (C) 2002 David C. Luff - david.luff@nottingham.ac.uk
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of the GNU General Public License as
|
|
// published by the Free Software Foundation; either version 2 of the
|
|
// License, or (at your option) any later version.
|
|
//
|
|
// This program is distributed in the hope that it will be useful, but
|
|
// WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU General Public License
|
|
// along with this program; if not, write to the Free Software
|
|
// Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
|
|
#ifndef _FG_AIEntity_HXX
|
|
#define _FG_AIEntity_HXX
|
|
|
|
#include <simgear/math/SGMath.hxx>
|
|
#include <simgear/scene/model/placement.hxx>
|
|
|
|
/*****************************************************************
|
|
*
|
|
* FGAIEntity - this class implements the minimum requirement
|
|
* for any AI entity - a position, an orientation, an associated
|
|
* 3D model, and the ability to be moved. It does nothing useful
|
|
* and all AI entities are expected to be derived from it.
|
|
*
|
|
******************************************************************/
|
|
class FGAIEntity {
|
|
|
|
public:
|
|
|
|
FGAIEntity();
|
|
virtual ~FGAIEntity();
|
|
|
|
// Set the 3D model to use (Must be called)
|
|
void SetModel(osg::Node* model);
|
|
|
|
// Run the internal calculations
|
|
virtual void Update(double dt)=0;
|
|
|
|
// Send a transmission *TO* the AIEntity.
|
|
// FIXME int code is a hack - eventually this will receive Alexander's coded messages.
|
|
virtual void RegisterTransmission(int code)=0;
|
|
|
|
const SGGeod& getPos() const
|
|
{ return _pos; }
|
|
|
|
virtual const string& GetCallsign()=0;
|
|
|
|
protected:
|
|
|
|
SGGeod _pos; // Geodetic position
|
|
double _hdg; //True heading in degrees
|
|
double _roll; //degrees
|
|
double _pitch; //degrees
|
|
|
|
SGModelPlacement _aip;
|
|
double _ground_elevation_m;
|
|
|
|
void Transform();
|
|
};
|
|
|
|
#endif // _FG_AIEntity_HXX
|
|
|