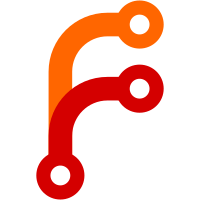
scene management code and organizing it within simgear. My strategy is to identify the code I want to move, and break it's direct flightgear dependencies. Then it will be free to move over into the simgear package. - Moved some property specific code into simgear/props/ - Split out the condition code from fgfs/src/Main/fg_props and put it in it's own source file in simgear/props/ - Created a scene subdirectory for scenery, model, and material property related code. - Moved location.[ch]xx into simgear/scene/model/ - The location and condition code had dependencies on flightgear's global state (all the globals-> stuff, the flightgear property tree, etc.) SimGear code can't depend on it so that data has to be passed as parameters to the functions/methods/constructors. - This need to pass data as function parameters had a dramatic cascading effect throughout the FlightGear code.
126 lines
3.1 KiB
C++
126 lines
3.1 KiB
C++
// model.cxx - manage a 3D aircraft model.
|
||
// Written by David Megginson, started 2002.
|
||
//
|
||
// This file is in the Public Domain, and comes with no warranty.
|
||
|
||
#ifdef HAVE_CONFIG_H
|
||
# include <config.h>
|
||
#endif
|
||
|
||
#include <string.h> // for strcmp()
|
||
|
||
#include <plib/sg.h>
|
||
#include <plib/ssg.h>
|
||
|
||
#include <simgear/compiler.h>
|
||
#include <simgear/debug/logstream.hxx>
|
||
#include <simgear/misc/exception.hxx>
|
||
#include <simgear/misc/sg_path.hxx>
|
||
|
||
#include <Main/globals.hxx>
|
||
#include <Main/fg_props.hxx>
|
||
#include <Main/viewmgr.hxx>
|
||
#include <Scenery/scenery.hxx>
|
||
|
||
#include "acmodel.hxx"
|
||
#include "model.hxx"
|
||
|
||
|
||
|
||
////////////////////////////////////////////////////////////////////////
|
||
// Implementation of FGAircraftModel
|
||
////////////////////////////////////////////////////////////////////////
|
||
|
||
FGAircraftModel::FGAircraftModel ()
|
||
: _aircraft(0),
|
||
_selector(new ssgSelector),
|
||
_scene(new ssgRoot),
|
||
_nearplane(0.01f),
|
||
_farplane(100.0f)
|
||
{
|
||
}
|
||
|
||
FGAircraftModel::~FGAircraftModel ()
|
||
{
|
||
delete _aircraft;
|
||
delete _scene;
|
||
// SSG will delete it
|
||
globals->get_scenery()->get_aircraft_branch()->removeKid(_selector);
|
||
}
|
||
|
||
void
|
||
FGAircraftModel::init ()
|
||
{
|
||
_aircraft = new FGModelPlacement;
|
||
string path = fgGetString("/sim/model/path", "Models/Geometry/glider.ac");
|
||
try {
|
||
_aircraft->init( globals->get_fg_root(),
|
||
path,
|
||
globals->get_props(),
|
||
globals->get_sim_time_sec() );
|
||
} catch (const sg_exception &ex) {
|
||
SG_LOG(SG_GENERAL, SG_ALERT, "Failed to load aircraft from " << path);
|
||
SG_LOG(SG_GENERAL, SG_ALERT, "(Falling back to glider.ac.)");
|
||
_aircraft->init( globals->get_fg_root(),
|
||
"Models/Geometry/glider.ac",
|
||
globals->get_props(),
|
||
globals->get_sim_time_sec() );
|
||
}
|
||
_scene->addKid(_aircraft->getSceneGraph());
|
||
_selector->addKid(_aircraft->getSceneGraph());
|
||
globals->get_scenery()->get_aircraft_branch()->addKid(_selector);
|
||
}
|
||
|
||
void
|
||
FGAircraftModel::bind ()
|
||
{
|
||
// No-op
|
||
}
|
||
|
||
void
|
||
FGAircraftModel::unbind ()
|
||
{
|
||
// No-op
|
||
}
|
||
|
||
void
|
||
FGAircraftModel::update (double dt)
|
||
{
|
||
int view_number = globals->get_viewmgr()->get_current();
|
||
|
||
if (view_number == 0 && !fgGetBool("/sim/view/internal")) {
|
||
_aircraft->setVisible(false);
|
||
} else {
|
||
_aircraft->setVisible(true);
|
||
}
|
||
|
||
_aircraft->setPosition(fgGetDouble("/position/longitude-deg"),
|
||
fgGetDouble("/position/latitude-deg"),
|
||
fgGetDouble("/position/altitude-ft"));
|
||
_aircraft->setOrientation(fgGetDouble("/orientation/roll-deg"),
|
||
fgGetDouble("/orientation/pitch-deg"),
|
||
fgGetDouble("/orientation/heading-deg"));
|
||
_aircraft->update( globals->get_scenery()->get_center() );
|
||
|
||
}
|
||
|
||
void
|
||
FGAircraftModel::draw ()
|
||
{
|
||
// OK, now adjust the clip planes and draw
|
||
// FIXME: view number shouldn't be
|
||
// hard-coded.
|
||
int view_number = globals->get_viewmgr()->get_current();
|
||
if (_aircraft->getVisible() && view_number == 0) {
|
||
glClearDepth(1);
|
||
glClear(GL_DEPTH_BUFFER_BIT);
|
||
ssgSetNearFar(_nearplane, _farplane);
|
||
ssgCullAndDraw(_scene);
|
||
_selector->select(0);
|
||
} else {
|
||
_selector->select(1);
|
||
}
|
||
|
||
}
|
||
|
||
// end of model.cxx
|