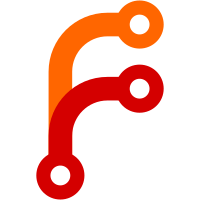
through the controls interface and the running and cranking flags through the engine interface. This has no current effect on LaRCsim (other than to make the code neater) but is necessary to add engine startup to JSBSim which is now underway. I've also put in main.cxx which escaped getting committed in the previous round of changes - adding this will add the cranking sound to LaRCsim during engine startup.
170 lines
4.7 KiB
C++
170 lines
4.7 KiB
C++
// controls.cxx -- defines a standard interface to all flight sim controls
|
|
//
|
|
// Written by Curtis Olson, started May 1997.
|
|
//
|
|
// Copyright (C) 1997 Curtis L. Olson - curt@infoplane.com
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of the GNU General Public License as
|
|
// published by the Free Software Foundation; either version 2 of the
|
|
// License, or (at your option) any later version.
|
|
//
|
|
// This program is distributed in the hope that it will be useful, but
|
|
// WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU General Public License
|
|
// along with this program; if not, write to the Free Software
|
|
// Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
//
|
|
// $Id$
|
|
|
|
|
|
#include "controls.hxx"
|
|
|
|
#include <simgear/debug/logstream.hxx>
|
|
#include <Main/fg_props.hxx>
|
|
|
|
|
|
// Constructor
|
|
FGControls::FGControls() :
|
|
aileron( 0.0 ),
|
|
elevator( 0.0 ),
|
|
elevator_trim( 1.969572E-03 ),
|
|
rudder( 0.0 ),
|
|
throttle_idle( true )
|
|
{
|
|
}
|
|
|
|
|
|
void FGControls::reset_all()
|
|
{
|
|
set_aileron(0.0);
|
|
set_elevator(0.0);
|
|
set_elevator_trim(0.0);
|
|
set_rudder(0.0);
|
|
set_throttle(FGControls::ALL_ENGINES, 0.0);
|
|
set_starter(FGControls::ALL_ENGINES, false);
|
|
set_magnetos(FGControls::ALL_ENGINES, 0);
|
|
throttle_idle = true;
|
|
gear_down = true;
|
|
}
|
|
|
|
|
|
// Destructor
|
|
FGControls::~FGControls() {
|
|
}
|
|
|
|
|
|
void
|
|
FGControls::init ()
|
|
{
|
|
for ( int engine = 0; engine < MAX_ENGINES; engine++ ) {
|
|
throttle[engine] = 0.0;
|
|
mixture[engine] = 1.0;
|
|
prop_advance[engine] = 1.0;
|
|
magnetos[engine] = 0;
|
|
starter[engine] = false;
|
|
}
|
|
|
|
for ( int wheel = 0; wheel < MAX_WHEELS; wheel++ ) {
|
|
brake[wheel] = 0.0;
|
|
}
|
|
|
|
auto_coordination = fgGetNode("/sim/auto-coordination", true);
|
|
}
|
|
|
|
|
|
void
|
|
FGControls::bind ()
|
|
{
|
|
fgTie("/controls/aileron", this,
|
|
&FGControls::get_aileron, &FGControls::set_aileron);
|
|
fgSetArchivable("/controls/aileron");
|
|
fgTie("/controls/elevator", this,
|
|
&FGControls::get_elevator, &FGControls::set_elevator);
|
|
fgSetArchivable("/controls/elevator");
|
|
fgTie("/controls/elevator-trim", this,
|
|
&FGControls::get_elevator_trim, &FGControls::set_elevator_trim);
|
|
fgSetArchivable("/controls/elevator-trim");
|
|
fgTie("/controls/rudder", this,
|
|
&FGControls::get_rudder, &FGControls::set_rudder);
|
|
fgSetArchivable("/controls/rudder");
|
|
fgTie("/controls/flaps", this,
|
|
&FGControls::get_flaps, &FGControls::set_flaps);
|
|
fgSetArchivable("/controls/flaps");
|
|
int index;
|
|
for (index = 0; index < MAX_ENGINES; index++) {
|
|
char name[32];
|
|
sprintf(name, "/controls/throttle[%d]", index);
|
|
fgTie(name, this, index,
|
|
&FGControls::get_throttle, &FGControls::set_throttle);
|
|
fgSetArchivable(name);
|
|
sprintf(name, "/controls/mixture[%d]", index);
|
|
fgTie(name, this, index,
|
|
&FGControls::get_mixture, &FGControls::set_mixture);
|
|
fgSetArchivable(name);
|
|
sprintf(name, "/controls/propellor-pitch[%d]", index);
|
|
fgTie(name, this, index,
|
|
&FGControls::get_prop_advance, &FGControls::set_prop_advance);
|
|
fgSetArchivable(name);
|
|
sprintf(name, "/controls/magnetos[%d]", index);
|
|
fgTie(name, this, index,
|
|
&FGControls::get_magnetos, &FGControls::set_magnetos);
|
|
fgSetArchivable(name);
|
|
sprintf(name, "/controls/starter[%d]", index);
|
|
fgTie(name, this, index,
|
|
&FGControls::get_starter, &FGControls::set_starter);
|
|
fgSetArchivable(name);
|
|
}
|
|
for (index = 0; index < MAX_WHEELS; index++) {
|
|
char name[32];
|
|
sprintf(name, "/controls/brakes[%d]", index);
|
|
fgTie(name, this, index,
|
|
&FGControls::get_brake, &FGControls::set_brake);
|
|
fgSetArchivable(name);
|
|
}
|
|
fgTie("/controls/gear-down", this,
|
|
&FGControls::get_gear_down, &FGControls::set_gear_down);
|
|
fgSetArchivable("/controls/gear-down");
|
|
}
|
|
|
|
|
|
void
|
|
FGControls::unbind ()
|
|
{
|
|
// Tie control properties.
|
|
fgUntie("/controls/aileron");
|
|
fgUntie("/controls/elevator");
|
|
fgUntie("/controls/elevator-trim");
|
|
fgUntie("/controls/rudder");
|
|
fgUntie("/controls/flaps");
|
|
int index;
|
|
for (index = 0; index < MAX_ENGINES; index++) {
|
|
char name[32];
|
|
sprintf(name, "/controls/throttle[%d]", index);
|
|
fgUntie(name);
|
|
sprintf(name, "/controls/mixture[%d]", index);
|
|
fgUntie(name);
|
|
sprintf(name, "/controls/propellor-pitch[%d]", index);
|
|
fgUntie(name);
|
|
sprintf(name, "/controls/magnetos[%d]", index);
|
|
fgUntie(name);
|
|
sprintf(name, "/controls/starter[%d]", index);
|
|
fgUntie(name);
|
|
}
|
|
for (index = 0; index < MAX_WHEELS; index++) {
|
|
char name[32];
|
|
sprintf(name, "/controls/brakes[%d]", index);
|
|
fgUntie(name);
|
|
}
|
|
fgUntie("/controls/gear-down");
|
|
}
|
|
|
|
|
|
void
|
|
FGControls::update ()
|
|
{
|
|
}
|
|
|