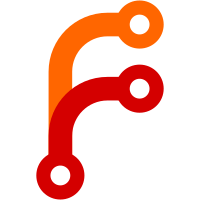
Change fgcommand to take an optional property tree root element. This fixes the animation bindings to use the defined property tree root - to support multiplayer (or other) model that can bind to the correct part of the property tree. Requires a corresponding fix in sg to allow the command methods to take an optional root parameter. What this means is that when inside someone else's multiplayer model (e.g. backseat, or co-pilot), the multipalyer (AI) model will correctly modify properties inside the correct part of the property tree inside (/ai), rather than modifying the properties inside the same part of the tree as the non-ai model. This means that a properly setup model will operate within it's own space in the property tree; and permit more generic multiplayer code to be written. This is probably responsible for some of the pollution of the root property tree with MP aircraft properties.
214 lines
5.8 KiB
C++
214 lines
5.8 KiB
C++
// route_mgr.hxx - manage a route (i.e. a collection of waypoints)
|
|
//
|
|
// Written by Curtis Olson, started January 2004.
|
|
//
|
|
// Copyright (C) 2004 Curtis L. Olson - http://www.flightgear.org/~curt
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of the GNU General Public License as
|
|
// published by the Free Software Foundation; either version 2 of the
|
|
// License, or (at your option) any later version.
|
|
//
|
|
// This program is distributed in the hope that it will be useful, but
|
|
// WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU General Public License
|
|
// along with this program; if not, write to the Free Software
|
|
// Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
//
|
|
// $Id$
|
|
|
|
|
|
#ifndef _ROUTE_MGR_HXX
|
|
#define _ROUTE_MGR_HXX 1
|
|
|
|
#include <simgear/props/props.hxx>
|
|
#include <simgear/structure/subsystem_mgr.hxx>
|
|
|
|
#include <Navaids/FlightPlan.hxx>
|
|
|
|
// forward decls
|
|
class SGPath;
|
|
class PropertyWatcher;
|
|
|
|
/**
|
|
* Top level route manager class
|
|
*
|
|
*/
|
|
|
|
class FGRouteMgr : public SGSubsystem,
|
|
public flightgear::FlightPlan::Delegate
|
|
{
|
|
public:
|
|
FGRouteMgr();
|
|
~FGRouteMgr();
|
|
|
|
void init ();
|
|
void postinit ();
|
|
void bind ();
|
|
void unbind ();
|
|
void update (double dt);
|
|
|
|
bool isRouteActive() const;
|
|
|
|
int currentIndex() const;
|
|
|
|
void setFlightPlan(const flightgear::FlightPlanRef& plan);
|
|
flightgear::FlightPlanRef flightPlan() const;
|
|
|
|
void clearRoute();
|
|
|
|
flightgear::Waypt* currentWaypt() const;
|
|
|
|
int numLegs() const;
|
|
|
|
// deprecated
|
|
int numWaypts() const
|
|
{ return numLegs(); }
|
|
|
|
// deprecated
|
|
flightgear::Waypt* wayptAtIndex(int index) const;
|
|
|
|
SGPropertyNode_ptr wayptNodeAtIndex(int index) const;
|
|
|
|
void removeLegAtIndex(int aIndex);
|
|
|
|
/**
|
|
* Activate a built route. This checks for various mandatory pieces of
|
|
* data, such as departure and destination airports, and creates waypoints
|
|
* for them on the route structure.
|
|
*
|
|
* returns true if the route was activated successfully, or false if the
|
|
* route could not be activated for some reason
|
|
*/
|
|
bool activate();
|
|
|
|
/**
|
|
* deactivate the route if active
|
|
*/
|
|
void deactivate();
|
|
|
|
/**
|
|
* Set the current waypoint to the specified index.
|
|
*/
|
|
void jumpToIndex(int index);
|
|
|
|
bool saveRoute(const SGPath& p);
|
|
bool loadRoute(const SGPath& p);
|
|
|
|
flightgear::WayptRef waypointFromString(const std::string& target);
|
|
|
|
static const char* subsystemName() { return "route-manager"; }
|
|
private:
|
|
bool commandDefineUserWaypoint(const SGPropertyNode * arg, SGPropertyNode * root);
|
|
bool commandDeleteUserWaypoint(const SGPropertyNode * arg, SGPropertyNode * root);
|
|
|
|
flightgear::FlightPlanRef _plan;
|
|
|
|
time_t _takeoffTime;
|
|
time_t _touchdownTime;
|
|
|
|
// automatic inputs
|
|
SGPropertyNode_ptr magvar;
|
|
|
|
// automatic outputs
|
|
SGPropertyNode_ptr departure; ///< departure airport information
|
|
SGPropertyNode_ptr destination; ///< destination airport information
|
|
SGPropertyNode_ptr alternate; ///< alternate airport information
|
|
SGPropertyNode_ptr cruise; ///< cruise information
|
|
|
|
SGPropertyNode_ptr totalDistance;
|
|
SGPropertyNode_ptr distanceToGo;
|
|
SGPropertyNode_ptr ete;
|
|
SGPropertyNode_ptr elapsedFlightTime;
|
|
|
|
SGPropertyNode_ptr active;
|
|
SGPropertyNode_ptr airborne;
|
|
|
|
SGPropertyNode_ptr wp0;
|
|
SGPropertyNode_ptr wp1;
|
|
SGPropertyNode_ptr wpn;
|
|
|
|
|
|
SGPropertyNode_ptr _pathNode;
|
|
SGPropertyNode_ptr _currentWpt;
|
|
|
|
|
|
/**
|
|
* Signal property to notify people that the route was edited
|
|
*/
|
|
SGPropertyNode_ptr _edited;
|
|
|
|
/**
|
|
* Signal property to notify when the last waypoint is reached
|
|
*/
|
|
SGPropertyNode_ptr _finished;
|
|
|
|
SGPropertyNode_ptr _flightplanChanged;
|
|
|
|
void setETAPropertyFromDistance(SGPropertyNode_ptr aProp, double aDistance);
|
|
|
|
/**
|
|
* retrieve the cached path distance along a leg
|
|
*/
|
|
double cachedLegPathDistanceM(int index) const;
|
|
double cachedWaypointPathTotalDistance(int index) const;
|
|
|
|
class InputListener : public SGPropertyChangeListener {
|
|
public:
|
|
InputListener(FGRouteMgr *m) : mgr(m) {}
|
|
virtual void valueChanged (SGPropertyNode * prop);
|
|
private:
|
|
FGRouteMgr *mgr;
|
|
};
|
|
|
|
SGPropertyNode_ptr input;
|
|
SGPropertyNode_ptr weightOnWheels;
|
|
SGPropertyNode_ptr groundSpeed;
|
|
|
|
InputListener *listener;
|
|
SGPropertyNode_ptr mirror;
|
|
|
|
/**
|
|
* Helper to keep various pieces of state in sync when the route is
|
|
* modified (waypoints added, inserted, removed). Notably, this fires the
|
|
* 'edited' signal.
|
|
*/
|
|
virtual void waypointsChanged();
|
|
|
|
void update_mirror();
|
|
|
|
virtual void currentWaypointChanged();
|
|
|
|
// tied getters and setters
|
|
std::string getDepartureICAO() const;
|
|
std::string getDepartureName() const;
|
|
void setDepartureICAO(const std::string& aIdent);
|
|
|
|
std::string getDepartureRunway() const;
|
|
void setDepartureRunway(const std::string& aIdent);
|
|
|
|
std::string getSID() const;
|
|
void setSID(const std::string& aIdent);
|
|
|
|
std::string getDestinationICAO() const;
|
|
std::string getDestinationName() const;
|
|
void setDestinationICAO(const std::string& aIdent);
|
|
|
|
std::string getDestinationRunway() const;
|
|
void setDestinationRunway(const std::string& aIdent);
|
|
|
|
std::string getApproach() const;
|
|
void setApproach(const std::string& aIdent);
|
|
|
|
std::string getSTAR() const;
|
|
void setSTAR(const std::string& aIdent);
|
|
|
|
double getDepartureFieldElevation() const;
|
|
double getDestinationFieldElevation() const;
|
|
};
|
|
|
|
|
|
#endif // _ROUTE_MGR_HXX
|