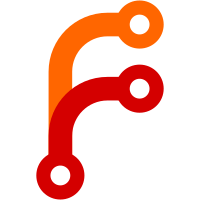
Clicking the close-button removes the widget from the sidebar Clicking the newwin-button detaches the widget into a dialog TODO: - add a way to re-attach or re-create a widget - make sure widgets correctly scale with resizing of the dialog - make widget area layout persistent
47 lines
1.2 KiB
JavaScript
47 lines
1.2 KiB
JavaScript
define([
|
|
'jquery', 'knockout', 'text!./sidebarwidget.html', 'jquery-ui/draggable', 'jquery-ui/dialog'
|
|
], function(jquery, ko, htmlString) {
|
|
|
|
function ViewModel(params, componentInfo) {
|
|
var self = this;
|
|
|
|
self.element = componentInfo.element;
|
|
|
|
self.widget = ko.observable(params.widget);
|
|
|
|
self.pinned = ko.observable(true);
|
|
self.pin = function() {
|
|
self.pinned(!self.pinned());
|
|
}
|
|
|
|
self.close = function() {
|
|
jquery(self.element).remove();
|
|
}
|
|
|
|
self.detach = function() {
|
|
jquery(self.element).find('.phi-widget').dialog();
|
|
jquery(self.element).remove();
|
|
}
|
|
|
|
self.expanded = ko.observable(true);
|
|
|
|
self.onMouseover = function() {
|
|
self.expanded(true);
|
|
}
|
|
|
|
self.onMouseout = function() {
|
|
if (!self.pinned())
|
|
self.expanded(false);
|
|
}
|
|
}
|
|
|
|
// Return component definition
|
|
return {
|
|
viewModel : {
|
|
createViewModel : function(params, componentInfo) {
|
|
return new ViewModel(params, componentInfo);
|
|
},
|
|
},
|
|
template : htmlString
|
|
};
|
|
});
|